πͺ Melee
A Melee represents an Entity which can be Pickable by a Character and can be used to melee attack, Charactes can hold it with hands with pre-defined handling modes.
πAuthority
This class can only be spawned on π¦ Server side.
πͺInheritance
π§βπ»API Source
This page is auto-generated! The Functions, Properties and Events described here are defined in our GitHub's API Repository! Feel free to commit suggestions and changes to the source .json API files!
π Examplesβ
local crowbar = Melee(
Vector(-900, 185, 215),
Rotator(0, 90, 90),
"nanos-world::SM_Crowbar_01",
CollisionType.Normal,
true,
HandlingMode.SingleHandedMelee,
""
)
crowbar:SetScale(Vector(1.5, 1.5, 1.5))
crowbar:AddAnimationCharacterUse("nanos-world::AM_Mannequin_Melee_Slash_Attack")
crowbar:SetDamageSettings(0.3, 0.5)
crowbar:SetCooldown(1.0)
crowbar:SetBaseDamage(40)
π Constructorsβ
Default Constructor
No description provided
local my_melee = Melee(location, rotation, asset, collision_type?, gravity_enabled?, handling_mode?, crosshair_material?, can_use?)
Type | Name | Default | Description |
---|---|---|---|
Vector | location | Required parameter | No description provided |
Rotator | rotation | Required parameter | No description provided |
StaticMesh Reference | asset | Required parameter | No description provided |
CollisionType | collision_type | CollisionType.Auto | No description provided |
boolean | gravity_enabled | true | No description provided |
HandlingMode | handling_mode | HandlingMode.Torch | No description provided |
Material Reference | crosshair_material |
| No description provided |
boolean | can_use | true | No description provided |
tip
nanos world provides a special Particle* called nanos-world::P_Grenade_Special
which spawns different particles depending on the surface it explodes, and also if is underwater.
*This "Particle" is just a special identifier which can only be used on Grenades!
πΏ Static Functionsβ
Inherited Entity Static Functions
This class doesn't have own static functions.
π¦ Functionsβ
Inherited Entity Functions
Inherited Actor Functions
Inherited Paintable Functions
Inherited Pickable Functions
Returns | Name | Description | |
---|---|---|---|
AddAnimationCharacterUse | Sets an Animation when attacking | ||
ClearAnimationsCharacterUse | Clears the Character Attack Animation list | ||
![]() | table of Animation Reference | GetAnimationsCharacterUse | Gets the Animations when Character uses it |
![]() | integer | GetBaseDamage | Gets the Base Damage |
![]() | float | GetCooldown | Gets the Cooldown between usages |
![]() | Sound Reference | GetSoundUse | Gets the Sound when Character uses it |
SetBaseDamage | Sets the Base Damage | ||
![]() | SetCooldown | Sets the cooldown between attacking | |
SetDamageSettings | Sets the times when to start applying damage and when to end | ||
![]() | SetImpactSound | Sets the Sound when hitting something | |
![]() | SetSoundUse | Sets the Sound when attacking |

AddAnimationCharacterUse
Sets an Animation when attacking
You can add more than one animation, which will be selected randomly when attacking
my_melee:AddAnimationCharacterUse(asset_path, play_rate, slot_Type)
Type | Parameter | Default | Description |
---|---|---|---|
Animation Reference | asset_path | Required parameter | The Animation used when attacking |
float | play_rate | Required parameter | The Animation Play Rate |
AnimationSlotType | slot_Type | Required parameter | Whether to play it on upper body or full body |

ClearAnimationsCharacterUse
Clears the Character Attack Animation list
my_melee:ClearAnimationsCharacterUse()
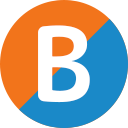
GetAnimationsCharacterUse
Gets the Animations when Character uses it
β Returns table of Animation Reference.
local ret = my_melee:GetAnimationsCharacterUse()
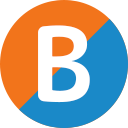
GetBaseDamage
Gets the Base Damage
β Returns integer.
local ret = my_melee:GetBaseDamage()
See also SetBaseDamage.
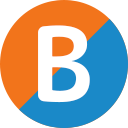
GetCooldown
Gets the Cooldown between usages
β Returns float.
local ret = my_melee:GetCooldown()
See also SetCooldown.
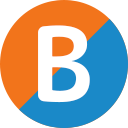
GetSoundUse
Gets the Sound when Character uses it
β Returns Sound Reference.
local ret = my_melee:GetSoundUse()
See also SetSoundUse.

SetBaseDamage
Sets the Base Damage
my_melee:SetBaseDamage(damage?)
Type | Parameter | Default | Description |
---|---|---|---|
integer | damage? |
| The Base Damage value |
See also GetBaseDamage.
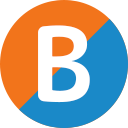
SetCooldown
Sets the cooldown between attacking
my_melee:SetCooldown(cooldown)
Type | Parameter | Default | Description |
---|---|---|---|
float | cooldown | Required parameter | No description provided |
See also GetCooldown.

SetDamageSettings
Sets the times when to start applying damage and when to end. During this time the collision of the melee will be enabled and the damage will be applied if it hits something
my_melee:SetDamageSettings(damage_start_time, damage_duration_time)
Type | Parameter | Default | Description |
---|---|---|---|
float | damage_start_time | Required parameter | The initial time to start applying damage |
float | damage_duration_time | Required parameter | The duration time to stop applying damage |
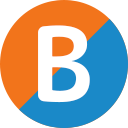
SetImpactSound
Sets the Sound when hitting something
Note: Surfaces Water and Flesh already have default sounds and must be explicitly set to override
my_melee:SetImpactSound(surface_type, asset_path, volume, pitch)
Type | Parameter | Default | Description |
---|---|---|---|
SurfaceType | surface_type | Required parameter | The surface to apply the sound. Use SurfaceType.Default to be the default to all hits |
Sound Reference | asset_path | Required parameter | The Sound used when attacking |
float | volume | Required parameter | No description provided |
float | pitch | Required parameter | No description provided |
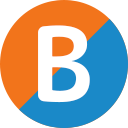
SetSoundUse
Sets the Sound when attacking
my_melee:SetSoundUse(asset_path)
Type | Parameter | Default | Description |
---|---|---|---|
Sound Reference | asset_path | Required parameter | The Sound used when attacking |
See also GetSoundUse.
π Eventsβ
Inherited Entity Events
Inherited Actor Events
Inherited Pickable Events
Name | Description | |
---|---|---|
![]() | Attack | Triggered when the Character effectively attacks with this Melee |
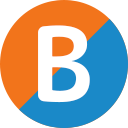
Attack
Triggered when the Character effectively attacks with this Melee
Melee.Subscribe("Attack", function(self, handler)
-- Attack was called
end)