🪤 Trigger
A Trigger class is a utility class to trigger events when any Entity enters an Area.
💂Authority
👪Inheritance
This class shares methods and events from Base Entity, Base Actor.
🧑💻API Source
This page is auto-generated! The Functions, Properties and Events described here are defined in our GitHub's API Repository! Feel free to commit suggestions and changes to the source .json API files!
🎒 Examples
local sphere_trigger = Trigger(Vector(-200, 100, 500), Rotator(), Vector(100), TriggerType.Sphere, true, Color(1, 0, 0))
sphere_trigger:Subscribe("BeginOverlap", function(trigger, actor_triggering)
Console.Log("Something entered my Sphere Trigger")
end)
local box_trigger = Trigger(Vector(300, 200, 500), Rotator(0, 45, 0), Vector(150, 150, 150), TriggerType.Box, true, Color(0, 1, 0))
box_trigger:Subscribe("BeginOverlap", function(trigger, actor_triggering)
Console.Log("Something entered my Box Trigger")
end)
box_trigger:Subscribe("EndOverlap", function(trigger, actor_triggering)
Console.Log("Something left my Box Trigger")
end)
(Trigger.SetOverlapOnlyClasses) Sets to only overlap Characters
my_trigger:SetOverlapOnlyClasses({ "Character", "CharacterSimple" })
🛠 Constructors
Default Constructor
local my_trigger = Trigger(location, rotation, extent, trigger_type?, is_visible?, color?, overlap_only_classes?)
Type | Name | Default | Description |
---|---|---|---|
Vector | location | Required parameter | No description provided |
Rotator | rotation | Required parameter | No description provided |
Vector or float | extent | Required parameter | Size of the Trigger. If using TriggerType.Sphere it is treated as radius, otherwise as Vector extent |
TriggerType | trigger_type | TriggerType.Sphere | No description provided |
boolean | is_visible | false | Useful for debugging |
Color | color | Color.RED | Color to paint the Trigger bounds - if Visible |
table of string | overlap_only_classes | {} | Filter Trigger to only overlap specific Classes. Leave it empty for all Classes |
🗿 Static Functions
Inherited Entity Static Functions
Trigger inherits from Base Entity Class, sharing it's methods and functions:
Base Entityscripting-reference/classes/base-classes/Entity
Base Entityscripting-reference/classes/base-classes/Entity
Returns | Name | Description | |
---|---|---|---|
![]() | table of Base Entity | GetAll | Returns a table containing all Entities of the class this is called on |
![]() | Base Entity | GetByIndex | Returns a specific Entity of this class at an index |
![]() | integer | GetCount | Returns how many Entities of this class exist |
![]() | table of table | GetInheritedClasses | Gets a list of all directly inherited classes from this Class created with the Inheriting System |
![]() | iterator | GetPairs | Returns an iterator with all Entities of this class to be used with pairs() |
![]() | table or nil | GetParentClass | Gets the parent class if this Class was created with the Inheriting System |
![]() | table | Inherit | Inherits this class with the Inheriting System |
![]() | boolean | IsChildOf | Gets if this Class is child of another class if this Class was created with the Inheriting System |
![]() | function | Subscribe | Subscribes to an Event for all entities of this Class |
![]() | function | SubscribeRemote | Subscribes to a custom event called from server |
![]() | Unsubscribe | Unsubscribes all callbacks from this Event in this Class within this Package, or only the callback passed |
🦠 Functions
Inherited Entity Functions
Trigger inherits from Base Entity Class, sharing it's methods and functions:
Base Entityscripting-reference/classes/base-classes/Entity
Base Entityscripting-reference/classes/base-classes/Entity
Returns | Name | Description | |
---|---|---|---|
BroadcastRemoteEvent | Calls a custom remote event directly on this entity to all Players | ||
CallRemoteEvent | Calls a custom remote event directly on this entity to a specific Player | ||
CallRemoteEvent | Calls a custom remote event directly on this entity | ||
Destroy | Destroys this Entity | ||
table of string | GetAllValuesKeys | Gets a list of all values keys | |
![]() | table | GetClass | Gets the class of this entity |
![]() | integer | GetID | Gets the universal network ID of this Entity (same on both client and server) |
![]() | any | GetValue | Gets a Value stored on this Entity at the given key |
![]() | boolean | IsA | Recursively checks if this entity is inherited from a Class |
![]() | boolean | IsValid | Returns true if this Entity is valid (i.e. wasn't destroyed and points to a valid Entity) |
![]() | SetValue | Sets a Value in this Entity | |
![]() | function | Subscribe | Subscribes to an Event on this specific entity |
![]() | function | SubscribeRemote | Subscribes to a custom event called from server on this specific entity |
![]() | Unsubscribe | Unsubscribes all callbacks from this Event in this Entity within this Package, or only the callback passed |
Inherited Actor Functions
Trigger inherits from Base Actor Class, sharing it's methods and functions:
Base Actorscripting-reference/classes/base-classes/Actor
Base Actorscripting-reference/classes/base-classes/Actor
Returns | Name | Description | |
---|---|---|---|
AddActorTag | Adds an Unreal Actor Tag to this Actor | ||
![]() | AddImpulse | Applies a force in world world to this Actor | |
AttachTo | Attaches this Actor to any other Actor, optionally at a specific bone | ||
Detach | Detaches this Actor from AttachedTo Actor | ||
table of string | GetActorTags | Gets all Unreal Actor Tags on this Actor | |
![]() | table of Base Actor | GetAttachedEntities | Gets all Actors attached to this Actor |
![]() | Base Actor or nil | GetAttachedTo | Gets the Actor this Actor is attached to |
table | GetBounds | Gets this Actor's bounds | |
![]() | CollisionType | GetCollision | Gets this Actor's collision type |
![]() | integer | GetDimension | Gets this Actor's dimension |
float | GetDistanceFromCamera | Gets the distance of this Actor from the Camera | |
![]() | Vector | GetForce | Gets this Actor's force (set by SetForce() ) |
![]() | Vector | GetLocation | Gets this Actor's location in the game world |
Player or nil | GetNetworkAuthority | Gets this Actor's Network Authority Player | |
![]() | Vector | GetRelativeLocation | Gets this Actor's Relative Location if it's attached |
![]() | Rotator | GetRelativeRotation | Gets this Actor's Relative Rotation if it's attached |
![]() | Rotator | GetRotation | Gets this Actor's angle in the game world |
![]() | Vector | GetScale | Gets this Actor's scale |
float | GetScreenPercentage | Gets the percentage of this Actor size in the screen | |
![]() | Vector | GetVelocity | Gets this Actor's current velocity |
boolean | HasAuthority | Gets if this Actor was spawned by the client side | |
boolean | HasNetworkAuthority | Returns true if the local Player is currently the Network Authority of this Actor | |
![]() | boolean | IsBeingDestroyed | Returns true if this Actor is being destroyed |
![]() | boolean | IsGravityEnabled | Returns true if gravity is enabled on this Actor |
![]() | boolean | IsInWater | Returns true if this Actor is in water |
![]() | boolean | IsNetworkDistributed | Returns true if this Actor is currently network distributed |
![]() | boolean | IsVisible | Returns true if this Actor is visible |
RemoveActorTag | Removes an Unreal Actor Tag from this Actor | ||
![]() | RotateTo | Smoothly rotates this actor to an angle over a certain time | |
SetCollision | Sets this Actor's collision type | ||
SetDimension | Sets this Actor's Dimension | ||
![]() | SetForce | Adds a permanent force to this Actor, set to Vector(0, 0, 0) to cancel | |
SetGravityEnabled | Sets whether gravity is enabled on this Actor | ||
SetHighlightEnabled | Sets whether the highlight is enabled on this Actor, and which highlight index to use | ||
SetLifeSpan | Sets the time (in seconds) before this Actor is destroyed. After this time has passed, the actor will be automatically destroyed. | ||
SetLocation | Sets this Actor's location in the game world | ||
SetNetworkAuthority | Sets the Player to have network authority over this Actor | ||
SetNetworkAuthorityAutoDistributed | Sets if this Actor will auto distribute the network authority between players | ||
SetOutlineEnabled | Sets whether the outline is enabled on this Actor, and which outline index to use | ||
SetRelativeLocation | Sets this Actor's relative location in local space (only if this actor is attached) | ||
SetRelativeRotation | Sets this Actor's relative rotation in local space (only if this actor is attached) | ||
SetRotation | Sets this Actor's rotation in the game world | ||
SetScale | Sets this Actor's scale | ||
![]() | SetVisibility | Sets whether the actor is visible or not | |
![]() | TranslateTo | Smoothly moves this actor to a location over a certain time | |
boolean | WasRecentlyRendered | Gets if this Actor was recently rendered on screen |
Returns | Name | Description | |
---|---|---|---|
ForceOverlapChecking | Forces a Overlap checking to occur, will immediately trigger overlaps | ||
![]() | SetColor | Sets the Trigger color (if visible) | |
SetExtent | Sets the extent size of this trigger (sphere triggers can receive as float for radius) | ||
SetOverlapOnlyClasses | Sets the filter to Trigger to only overlap specific Classes. Leave it empty for all Classes |

ForceOverlapChecking
Forces a Overlap checking to occur, will immediately trigger overlaps
my_trigger:ForceOverlapChecking()
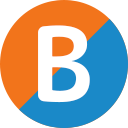
SetColor
Sets the Trigger color (if visible)
my_trigger:SetColor(color)
Type | Parameter | Default | Description |
---|---|---|---|
Color | color | Required parameter | No description provided |

SetExtent
Sets the extent size of this trigger (sphere triggers can receive as float for radius)
my_trigger:SetExtent(extent)

SetOverlapOnlyClasses
Sets the filter to Trigger to only overlap specific Classes. Leave it empty for all Classes
my_trigger:SetOverlapOnlyClasses(overlap_only_classes)
Type | Parameter | Default | Description |
---|---|---|---|
table of string | overlap_only_classes | Required parameter | No description provided |
Trigger.SetOverlapOnlyClasses Examples
Sets to only overlap Characters
my_trigger:SetOverlapOnlyClasses({ "Character", "CharacterSimple" })
🚀 Events
Inherited Entity Events
Trigger inherits from Base Entity Class, sharing it's events:
Base Entityscripting-reference/classes/base-classes/Entity
Base Entityscripting-reference/classes/base-classes/Entity
Name | Description | |
---|---|---|
![]() | ClassRegister | Triggered when a new Class is registered with the Inheriting System |
![]() | Destroy | Triggered when an Entity is destroyed |
![]() | Spawn | Triggered when an Entity is spawned/created |
![]() | ValueChange | Triggered when an Entity has a value changed with :SetValue() |
Inherited Actor Events
Trigger inherits from Base Actor Class, sharing it's events:
Base Actorscripting-reference/classes/base-classes/Actor
Base Actorscripting-reference/classes/base-classes/Actor
Name | Description | |
---|---|---|
DimensionChange | Triggered when an Actor changes it's dimension |
Name | Description | |
---|---|---|
BeginOverlap | Triggered when something overlaps this Trigger | |
EndOverlap | Triggered when something leaves this Trigger |

BeginOverlap
Triggered when something overlaps this Trigger
Trigger.Subscribe("BeginOverlap", function(self, entity)
-- BeginOverlap was called
end)
Type | Argument | Description |
---|---|---|
Trigger | self | The Trigger entity |
Base Actor | entity | Any Actor which overlaps |

EndOverlap
Triggered when something leaves this Trigger
Trigger.Subscribe("EndOverlap", function(self, entity)
-- EndOverlap was called
end)
Type | Argument | Description |
---|---|---|
Trigger | self | The Trigger entity |
Base Actor | entity | Any Actor which left the Trigger |