π§β𦲠CharacterSimple
CharacterSimple is a simpler Character implementation with basic Movement implementation. Aimed for custom NPCs or basic Pawns.
πAuthority
πͺInheritance
This class shares methods and events from Base Entity, Base Actor, Base Pawn, Base Paintable, Base Damageable.
π§βπ»API Source
This page is auto-generated! The Functions, Properties and Events described here are defined in our GitHub's API Repository! Feel free to commit suggestions and changes to the source .json API files!
π Examplesβ
Server/Index.lua
-- Spawns a Stack-O-Bot Character
local stack_o_bot = CharacterSimple(Vector(100, 0, 100), Rotator(0, 0, 0), "nanos-world::SK_StackOBot", "nanos-world::ABP_StackOBot")
stack_o_bot:SetSpeedSettings(275, 150)
π Constructorsβ
Default Constructor
No description provided
local my_charactersimple = CharacterSimple(location, rotation, mesh, custom_animation_blueprint?, collision_type?, gravity_enabled?)
Type | Name | Default | Description |
---|---|---|---|
Vector | location | Required parameter | No description provided |
Rotator | rotation | Required parameter | No description provided |
SkeletalMesh Reference or StaticMesh Reference | mesh | Required parameter | No description provided |
Blueprint Reference | custom_animation_blueprint |
| No description provided |
CollisionType | collision_type | CollisionType.Auto | No description provided |
boolean | gravity_enabled | true | No description provided |
πΏ Static Functionsβ
Inherited Entity Static Functions
This class doesn't have own static functions.
π¦ Functionsβ
Inherited Entity Functions
Inherited Actor Functions
Inherited Paintable Functions
Inherited Damageable Functions
Inherited Pawn Functions
Returns | Name | Description | |
---|---|---|---|
![]() | varargs of any | CallAnimationBlueprintEvent | Calls an Animation Blueprint Event or Function |
PlayAnimation | Plays an Animation Montage on this character | ||
SetAirControl | Sets the amount of movement control allowed when it is in air | ||
SetAnimationBlueprint | Sets the Animation Blueprint of this Character | ||
SetMaxAcceleration | Sets the max acceleration | ||
SetMesh | Changes the Character Mesh on the fly | ||
SetMesh | Changes the Character Mesh on the fly | ||
SetPawnSettings | Sets the Pawn Settings of this Character | ||
SetRotationSettings | Sets the Rotation Settings of this Character | ||
SetSpeedSettings | Sets the Speed Settings of this Character | ||
![]() | SetSpringArmSettings | Sets the Spring Arm Settings of this Character |
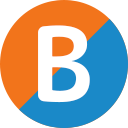
CallAnimationBlueprintEvent
Calls an Animation Blueprint Event or Function
Returns all Function return values on Client Side
β Returns varargs of any (the function return values).
local ret_01, ret_02, ... = my_charactersimple:CallAnimationBlueprintEvent(event_name, arguments...?)
Type | Parameter | Default | Description |
---|---|---|---|
string | event_name | Required parameter | Event or Function name |
any | arguments...? | nil | Sequence of arguments to pass to the event |

PlayAnimation
Plays an Animation Montage on this character
my_charactersimple:PlayAnimation(animation_path, slot_name?, loop_indefinitely?, blend_in_time?, blend_out_time?, play_rate?, stop_all_montages?)
Type | Parameter | Default | Description |
---|---|---|---|
Animation Reference | animation_path | Required parameter | No description provided |
string | slot_name? |
| No description provided |
boolean | loop_indefinitely? | false | No description provided |
float | blend_in_time? | 0.25 | No description provided |
float | blend_out_time? | 0.25 | No description provided |
float | play_rate? | 1.0 | No description provided |
boolean | stop_all_montages? | false | Stops all running Montages from the same Group |

SetAirControl
Sets the amount of movement control allowed when it is in air
my_charactersimple:SetAirControl(air_control?, boost_multiplier?, boost_velocity_threshold?)
Type | Parameter | Default | Description |
---|---|---|---|
float | air_control? | 0.2 | When falling, amount of lateral movement control available to the character. 0 = no control, 1 = full control at max speed of MaxWalkSpeed |
float | boost_multiplier? | 512 | When falling, multiplier applied to air_control when lateral velocity is less than boost_velocity_threshold. Setting this to zero will disable air control boosting. Final result is clamped at 1 |
float | boost_velocity_threshold? | 25 | When falling, if lateral velocity magnitude is less than this value, air_control is multiplied by boost_multiplier. Setting this to zero will disable air control boosting |

SetAnimationBlueprint
Sets the Animation Blueprint of this Character
my_charactersimple:SetAnimationBlueprint(custom_animation_blueprint)
Type | Parameter | Default | Description |
---|---|---|---|
Blueprint Reference | custom_animation_blueprint | Required parameter | No description provided |

SetMaxAcceleration
Sets the max acceleration
my_charactersimple:SetMaxAcceleration(acceleration)
Type | Parameter | Default | Description |
---|---|---|---|
integer | acceleration | Required parameter | Default is 2048 |

SetMesh
Changes the Character Mesh on the fly
my_charactersimple:SetMesh(mesh_asset, adjust_capsule_size)
Type | Parameter | Default | Description |
---|---|---|---|
SkeletalMesh Reference or StaticMesh Reference | mesh_asset | Required parameter | No description provided |
boolean | adjust_capsule_size | Required parameter | Auto adjust the capsule size based on the Mesh size |

SetMesh
Changes the Character Mesh on the fly
my_charactersimple:SetMesh(mesh, adjust_capsule_size)
Type | Parameter | Default | Description |
---|---|---|---|
SkeletalMesh Reference or StaticMesh Reference | mesh | Required parameter | No description provided |
boolean | adjust_capsule_size | Required parameter | Auto adjust the capsule size based on the Mesh size |

SetPawnSettings
Sets the Pawn Settings of this Character
my_charactersimple:SetPawnSettings(use_controller_rotation_pitch, use_controller_rotation_yaw, use_controller_rotation_roll)
Type | Parameter | Default | Description |
---|---|---|---|
boolean | use_controller_rotation_pitch | Required parameter | No description provided |
boolean | use_controller_rotation_yaw | Required parameter | No description provided |
boolean | use_controller_rotation_roll | Required parameter | No description provided |

SetRotationSettings
Sets the Rotation Settings of this Character
my_charactersimple:SetRotationSettings(rotation_rate, use_controller_desired_rotation, orient_rotation_to_movement)
Type | Parameter | Default | Description |
---|---|---|---|
Rotator | rotation_rate | Required parameter | No description provided |
boolean | use_controller_desired_rotation | Required parameter | No description provided |
boolean | orient_rotation_to_movement | Required parameter | No description provided |

SetSpeedSettings
Sets the Speed Settings of this Character
my_charactersimple:SetSpeedSettings(max_walk_speed, max_walk_speed_crouched)
Type | Parameter | Default | Description |
---|---|---|---|
integer | max_walk_speed | Required parameter | No description provided |
integer | max_walk_speed_crouched | Required parameter | No description provided |
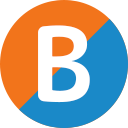
SetSpringArmSettings
Sets the Spring Arm Settings of this Character
my_charactersimple:SetSpringArmSettings(relative_location?, target_arm_length?, socket_offset?, enable_camera_lag?, camera_lag_speed?, camera_lag_max_distance?)
Type | Parameter | Default | Description |
---|---|---|---|
Vector | relative_location? | Vector(0, 0, 144) | No description provided |
float | target_arm_length? | 300.0 | No description provided |
Vector | socket_offset? | Vector(0, 0, 0) | No description provided |
boolean | enable_camera_lag? | true | No description provided |
float | camera_lag_speed? | 15.0 | No description provided |
float | camera_lag_max_distance? | 1.0 | No description provided |
π Eventsβ
Inherited Entity Events
Inherited Actor Events
Inherited Damageable Events
Inherited Pawn Events
This class doesn't have own events.