🚙 VehicleWheeled
Vehicles are wheeled entities which Characters can possesses and drive.
💂Authority
This class can only be spawned on 🟦 Server side.
👪Inheritance
This class shares methods and events from Base Entity, Base Actor, Base Vehicle, Base Paintable, Base Damageable.
🧑💻API Source
This page is auto-generated! The Functions, Properties and Events described here are defined in our GitHub's API Repository! Feel free to commit suggestions and changes to the source .json API files!
Any Skeletal Mesh can be used to create a Vehicle, although only Skeletal Meshes with Wheels bones can use the built-in feature of animated Wheels.
🎒 Examples
Server/Index.lua
-- Spawns a Pickup Vehicle
local vehicle = VehicleWheeled(location or Vector(), rotation or Rotator(), "nanos-world::SK_Pickup", CollisionType.Normal, true, false, true, "nanos-world::A_Vehicle_Engine_10")
-- Configure it's Engine power and Aerodynamics
vehicle:SetEngineSetup(700, 5000)
vehicle:SetAerodynamicsSetup(2500)
-- Configure it's Steering Wheel and Headlights location
vehicle:SetSteeringWheelSetup(Vector(0, 27, 120), 24)
vehicle:SetHeadlightsSetup(Vector(270, 0, 70))
-- Configures each Wheel
vehicle:SetWheel(0, "Wheel_Front_Left", 27, 18, 45, Vector(), true, true, false, false, false, 1500, 3000, 1000, 1, 3, 20, 20, 250, 50, 10, 10, 0, 0.5, 0.5)
vehicle:SetWheel(1, "Wheel_Front_Right", 27, 18, 45, Vector(), true, true, false, false, false, 1500, 3000, 1000, 1, 3, 20, 20, 250, 50, 10, 10, 0, 0.5, 0.5)
vehicle:SetWheel(2, "Wheel_Rear_Left", 27, 18, 0, Vector(), false, true, true, false, false, 1500, 3000, 1000, 1, 4, 20, 20, 250, 50, 10, 10, 0, 0.5, 0.5)
vehicle:SetWheel(3, "Wheel_Rear_Right", 27, 18, 0, Vector(), false, true, true, false, false, 1500, 3000, 1000, 1, 4, 20, 20, 250, 50, 10, 10, 0, 0.5, 0.5)
-- Adds 6 Doors/Seats
vehicle:SetDoor(0, Vector( 50, -75, 105), Vector( 8, -32.5, 95), Rotator(0, 0, 10), 70, -150)
vehicle:SetDoor(1, Vector( 50, 75, 105), Vector( 25, 50, 90), Rotator(0, 0, 0), 70, 150)
vehicle:SetDoor(2, Vector( -90, -75, 130), Vector( -90, -115, 155), Rotator(0, 90, 20), 60, -150)
vehicle:SetDoor(3, Vector( -90, 75, 130), Vector( -90, 115, 155), Rotator(0, -90, 20), 60, 150)
vehicle:SetDoor(4, Vector(-195, -75, 130), Vector(-195, -115, 155), Rotator(0, 90, 20), 60, -150)
vehicle:SetDoor(5, Vector(-195, 75, 130), Vector(-195, 115, 155), Rotator(0, -90, 20), 60, 150)
-- Make it ready (so clients only create Physics once and not for each function call above)
vehicle:RecreatePhysics()
🛠 Constructors
Default Constructor
local my_vehiclewheeled = VehicleWheeled(location, rotation, asset, collision_type?, gravity_enabled?, auto_create_physics?, auto_unflip?, engine_sound?, horn_sound?, brake_sound?, engine_start_sound?, vehicle_door_sound?, auto_start_engine?, custom_animation_blueprint?)
Type | Name | Default | Description |
---|---|---|---|
Vector | location | Required parameter | No description provided |
Rotator | rotation | Required parameter | No description provided |
SkeletalMesh Reference | asset | Required parameter | No description provided |
CollisionType | collision_type | CollisionType.Auto | No description provided |
boolean | gravity_enabled | true | No description provided |
boolean | auto_create_physics | true | Can be disabled to improve performance when setting several configs. Must call RecreatePhysics() after all |
boolean | auto_unflip | true | Auto rotates the vehicle if flipped |
Sound Reference | engine_sound | nanos-world::A_Vehicle_Engine_01 | No description provided |
Sound Reference | horn_sound | nanos-world::A_Vehicle_Horn_Toyota | No description provided |
Sound Reference | brake_sound | nanos-world::A_Vehicle_Brake | No description provided |
Sound Reference | engine_start_sound | nanos-world::A_Car_Engine_Start | No description provided |
Sound Reference | vehicle_door_sound | nanos-world::A_Vehicle_Door | No description provided |
boolean | auto_start_engine | true | No description provided |
Blueprint Reference | custom_animation_blueprint |
| No description provided |
tip
Please take a look at our Default's Vehicle package with all built-in Vehicles already properly configured and ready to use.
More related examples:
Monster Truckgetting-started/tutorials-and-examples/monster-truck🗿 Static Functions
Inherited Entity Static Functions
VehicleWheeled inherits from Base Entity Class, sharing it's methods and functions:
Base Entityscripting-reference/classes/base-classes/Entity
Base Entityscripting-reference/classes/base-classes/Entity
Returns | Name | Description | |
---|---|---|---|
![]() | table of Base Entity | GetAll | Returns a table containing all Entities of the class this is called on |
![]() | Base Entity | GetByIndex | Returns a specific Entity of this class at an index |
![]() | integer | GetCount | Returns how many Entities of this class exist |
![]() | table of table | GetInheritedClasses | Gets a list of all directly inherited classes from this Class created with the Inheriting System |
![]() | iterator | GetPairs | Returns an iterator with all Entities of this class to be used with pairs() |
![]() | table or nil | GetParentClass | Gets the parent class if this Class was created with the Inheriting System |
![]() | table | Inherit | Inherits this class with the Inheriting System |
![]() | boolean | IsChildOf | Gets if this Class is child of another class if this Class was created with the Inheriting System |
![]() | function | Subscribe | Subscribes to an Event for all entities of this Class |
![]() | function | SubscribeRemote | Subscribes to a custom event called from server |
![]() | Unsubscribe | Unsubscribes all callbacks from this Event in this Class within this Package, or only the callback passed |
🦠 Functions
Inherited Entity Functions
VehicleWheeled inherits from Base Entity Class, sharing it's methods and functions:
Base Entityscripting-reference/classes/base-classes/Entity
Base Entityscripting-reference/classes/base-classes/Entity
Returns | Name | Description | |
---|---|---|---|
BroadcastRemoteEvent | Calls a custom remote event directly on this entity to all Players | ||
CallRemoteEvent | Calls a custom remote event directly on this entity to a specific Player | ||
CallRemoteEvent | Calls a custom remote event directly on this entity | ||
Destroy | Destroys this Entity | ||
table of string | GetAllValuesKeys | Gets a list of all values keys | |
![]() | table | GetClass | Gets the class of this entity |
![]() | integer | GetID | Gets the universal network ID of this Entity (same on both client and server) |
![]() | any | GetValue | Gets a Value stored on this Entity at the given key |
![]() | boolean | IsA | Recursively checks if this entity is inherited from a Class |
![]() | boolean | IsValid | Returns true if this Entity is valid (i.e. wasn't destroyed and points to a valid Entity) |
![]() | SetValue | Sets a Value in this Entity | |
![]() | function | Subscribe | Subscribes to an Event on this specific entity |
![]() | function | SubscribeRemote | Subscribes to a custom event called from server on this specific entity |
![]() | Unsubscribe | Unsubscribes all callbacks from this Event in this Entity within this Package, or only the callback passed |
Inherited Actor Functions
VehicleWheeled inherits from Base Actor Class, sharing it's methods and functions:
Base Actorscripting-reference/classes/base-classes/Actor
Base Actorscripting-reference/classes/base-classes/Actor
Returns | Name | Description | |
---|---|---|---|
AddActorTag | Adds an Unreal Actor Tag to this Actor | ||
![]() | AddImpulse | Applies a force in world world to this Actor | |
AttachTo | Attaches this Actor to any other Actor, optionally at a specific bone | ||
Detach | Detaches this Actor from AttachedTo Actor | ||
table of string | GetActorTags | Gets all Unreal Actor Tags on this Actor | |
![]() | table of Base Actor | GetAttachedEntities | Gets all Actors attached to this Actor |
![]() | Base Actor or nil | GetAttachedTo | Gets the Actor this Actor is attached to |
table | GetBounds | Gets this Actor's bounds | |
![]() | CollisionType | GetCollision | Gets this Actor's collision type |
![]() | integer | GetDimension | Gets this Actor's dimension |
float | GetDistanceFromCamera | Gets the distance of this Actor from the Camera | |
![]() | Vector | GetForce | Gets this Actor's force (set by SetForce() ) |
![]() | Vector | GetLocation | Gets this Actor's location in the game world |
Player or nil | GetNetworkAuthority | Gets this Actor's Network Authority Player | |
![]() | Vector | GetRelativeLocation | Gets this Actor's Relative Location if it's attached |
![]() | Rotator | GetRelativeRotation | Gets this Actor's Relative Rotation if it's attached |
![]() | Rotator | GetRotation | Gets this Actor's angle in the game world |
![]() | Vector | GetScale | Gets this Actor's scale |
float | GetScreenPercentage | Gets the percentage of this Actor size in the screen | |
![]() | Vector | GetVelocity | Gets this Actor's current velocity |
boolean | HasAuthority | Gets if this Actor was spawned by the client side | |
boolean | HasNetworkAuthority | Returns true if the local Player is currently the Network Authority of this Actor | |
![]() | boolean | IsBeingDestroyed | Returns true if this Actor is being destroyed |
![]() | boolean | IsGravityEnabled | Returns true if gravity is enabled on this Actor |
![]() | boolean | IsInWater | Returns true if this Actor is in water |
![]() | boolean | IsNetworkDistributed | Returns true if this Actor is currently network distributed |
![]() | boolean | IsVisible | Returns true if this Actor is visible |
RemoveActorTag | Removes an Unreal Actor Tag from this Actor | ||
![]() | RotateTo | Smoothly rotates this actor to an angle over a certain time | |
SetCollision | Sets this Actor's collision type | ||
SetDimension | Sets this Actor's Dimension | ||
![]() | SetForce | Adds a permanent force to this Actor, set to Vector(0, 0, 0) to cancel | |
SetGravityEnabled | Sets whether gravity is enabled on this Actor | ||
SetHighlightEnabled | Sets whether the highlight is enabled on this Actor, and which highlight index to use | ||
SetLifeSpan | Sets the time (in seconds) before this Actor is destroyed. After this time has passed, the actor will be automatically destroyed. | ||
SetLocation | Sets this Actor's location in the game world | ||
SetNetworkAuthority | Sets the Player to have network authority over this Actor | ||
SetNetworkAuthorityAutoDistributed | Sets if this Actor will auto distribute the network authority between players | ||
SetOutlineEnabled | Sets whether the outline is enabled on this Actor, and which outline index to use | ||
SetRelativeLocation | Sets this Actor's relative location in local space (only if this actor is attached) | ||
SetRelativeRotation | Sets this Actor's relative rotation in local space (only if this actor is attached) | ||
SetRotation | Sets this Actor's rotation in the game world | ||
SetScale | Sets this Actor's scale | ||
![]() | SetVisibility | Sets whether the actor is visible or not | |
![]() | TranslateTo | Smoothly moves this actor to a location over a certain time | |
boolean | WasRecentlyRendered | Gets if this Actor was recently rendered on screen |
Inherited Paintable Functions
VehicleWheeled inherits from Base Paintable Class, sharing it's methods and functions:
Base Paintablescripting-reference/classes/base-classes/Paintable
Base Paintablescripting-reference/classes/base-classes/Paintable
Returns | Name | Description | |
---|---|---|---|
![]() | ResetMaterial | Resets the material from the specified index to the original one | |
![]() | SetMaterial | Sets the material at the specified index of this Actor | |
![]() | SetMaterialColorParameter | Sets a Color parameter in this Actor's material | |
SetMaterialFromCanvas | Sets the material at the specified index of this Actor to a Canvas object | ||
SetMaterialFromSceneCapture | Sets the material at the specified index of this Actor to a SceneCapture object | ||
SetMaterialFromWebUI | Sets the material at the specified index of this Actor to a WebUI object | ||
![]() | SetMaterialScalarParameter | Sets a Scalar parameter in this Actor's material | |
![]() | SetMaterialTextureParameter | Sets a texture parameter in this Actor's material to an image on disk | |
![]() | SetMaterialVectorParameter | Sets a Vector parameter in this Actor's material | |
![]() | SetPhysicalMaterial | Overrides this Actor's Physical Material with a new one |
Inherited Damageable Functions
VehicleWheeled inherits from Base Damageable Class, sharing it's methods and functions:
Base Damageablescripting-reference/classes/base-classes/Damageable
Base Damageablescripting-reference/classes/base-classes/Damageable
Returns | Name | Description | |
---|---|---|---|
integer | ApplyDamage | Do damage to this entity | |
![]() | integer | GetHealth | Gets the current health |
![]() | integer | GetMaxHealth | Gets the Max Health |
Respawn | Respawns the Entity, fullying it's Health and moving it to it's Initial Location | ||
SetHealth | Sets the Health of this Entity | ||
SetMaxHealth | Sets the MaxHealth of this Entity |
Inherited Vehicle Functions
VehicleWheeled inherits from Base Vehicle Class, sharing it's methods and functions:
Base Vehiclescripting-reference/classes/base-classes/Vehicle
Base Vehiclescripting-reference/classes/base-classes/Vehicle
Returns | Name | Description | |
---|---|---|---|
![]() | AddSkeletalMeshAttached | Attaches a Skeletal Mesh as master pose to this entity | |
![]() | AddStaticMeshAttached | Attaches a Static Mesh to this entity | |
![]() | table of string | GetAllSkeletalMeshAttached | Gets all Skeletal Meshes attached to this entity |
![]() | table of string | GetAllStaticMeshAttached | Gets all Static Meshes attached to this entity |
table | GetBoneTransform | Gets a Bone Transform in world space given a bone name | |
![]() | table | GetDoors | Gets all configured Doors |
![]() | SkeletalMesh Reference | GetMesh | Gets the Asset name |
![]() | Character | GetPassenger | Gets a passenger from a seat |
![]() | table of Character | GetPassengers | Gets all passengers |
![]() | PlayAnimation | Plays an Animation on this Vehicle | |
![]() | RemoveAllSkeletalMeshesAttached | Removes all SkeletalMeshes attached | |
![]() | RemoveAllStaticMeshesAttached | Removes all StaticMeshes attached | |
![]() | RemoveSkeletalMeshAttached | Removes, if it exists, a SkeletalMesh from this Pickable given its custom ID | |
![]() | RemoveStaticMeshAttached | Removes, if it exists, a StaticMesh from this Pickable given its custom ID | |
SetDoor | Adds a Door to the Vehicle | ||
SetExplosionSettings | Configures the Explosion Settings when health reaches zero | ||
![]() | SetStaticMeshAttachedTransform | Sets a Static Mesh Attached location and rotation |
Returns | Name | Description | |
---|---|---|---|
integer | GetGear | Gets the current Gear | |
integer | GetRPM | Gets the current RPM | |
Horn | Starts or stops the vehicles horn | ||
RecreatePhysics | Recreate the Vehicle Physics | ||
SetAerodynamicsSetup | Configures the Vehicle Aerodynamics Settings | ||
SetAutoStartEngine | Sets if the Engine auto starts when the driver enters the Vehicle | ||
![]() | SetCameraOffset | Sets the Vehicle Camera Offset | |
SetEngineSetup | Configures the Vehicle Engine (Torque, RPM, Braking) | ||
SetEngineStarted | Sets if the Engine is turned off/on | ||
SetHeadlightsSetup | Configures the Headlights Offset and Color. | ||
SetSteeringSetup | Configures the Vehicle Steering | ||
SetSteeringWheelSetup | Configures where the Steering Wheel is located | ||
SetTaillightsSetup | Configures the Taillights Offset. | ||
SetTireFlat | Sets a Tire as Flat or not | ||
SetTransmissionSetup | Configures the Vehicle Transmission | ||
SetWheel | Configures a Vehicle Wheel |

GetGear
Gets the current Gear
— Returns integer.
local ret = my_vehiclewheeled:GetGear()

GetRPM
Gets the current RPM
— Returns integer.
local ret = my_vehiclewheeled:GetRPM()

Horn
Starts or stops the vehicles horn
my_vehiclewheeled:Horn(enable_horn)
Type | Parameter | Default | Description |
---|---|---|---|
boolean | enable_horn | Required parameter | No description provided |

RecreatePhysics
Call this after configuring the vehicle if passed auto_create_physics
to constructor
my_vehiclewheeled:RecreatePhysics()

SetAerodynamicsSetup
Configures the Vehicle Aerodynamics Settings
my_vehiclewheeled:SetAerodynamicsSetup(mass?, drag_coefficient?, vehicle_chassis_width?, vehicle_chassis_height?, vehicle_downforce_coefficient?, center_of_mass_override?)
Type | Parameter | Default | Description |
---|---|---|---|
integer | mass? | 1500 | Mass of the vehicle chassis |
float | drag_coefficient? | 0.3 | Force resisting forward motion at speed |
integer | vehicle_chassis_width? | 180 | Chassis width used for drag force computation (cm) |
integer | vehicle_chassis_height? | 140 | Chassis height used for drag force computation (cm) |
float | vehicle_downforce_coefficient? | 0.3 | Force pressing vehicle into ground at speed |
Vector | center_of_mass_override? | Vector(0, 0, 50) | Overrides the center of mass. Good for curves stabilization. Ideally the Z should be the same as the wheels radius |

SetAutoStartEngine
Sets if the Engine auto starts when the driver enters the Vehicle
my_vehiclewheeled:SetAutoStartEngine(auto_start)
Type | Parameter | Default | Description |
---|---|---|---|
boolean | auto_start | Required parameter | No description provided |
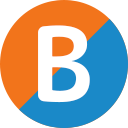
SetCameraOffset
Sets the Vehicle Camera Offset
my_vehiclewheeled:SetCameraOffset(offset)
Type | Parameter | Default | Description |
---|---|---|---|
Vector | offset | Required parameter | No description provided |

SetEngineSetup
Configures the Vehicle Engine (Torque, RPM, Braking)
my_vehiclewheeled:SetEngineSetup(max_torque?, max_rpm?, idle_rpm?, brake_effect?, rev_up_moi?, rev_down_rate?)
Type | Parameter | Default | Description |
---|---|---|---|
integer | max_torque? | 700 | Max Engine Torque (Nm) is multiplied by TorqueCurve |
integer | max_rpm? | 5700 | Maximum revolutions per minute of the engine |
integer | idle_rpm? | 1200 | Idle RMP of engine then in neutral/stationary |
float | brake_effect? | 0.05 | Braking effect from engine, when throttle released |
integer | rev_up_moi? | 5 | Affects how fast the engine RPM speed up |
integer | rev_down_rate? | 600 | Affects how fast the engine RPM slows down |

SetEngineStarted
Sets if the Engine is turned off/on (this will affect Lights, Sounds and ability to Throttle)
my_vehiclewheeled:SetEngineStarted(started)
Type | Parameter | Default | Description |
---|---|---|---|
boolean | started | Required parameter | No description provided |

SetHeadlightsSetup
Configures the Headlights Offset and Color.
my_vehiclewheeled:SetHeadlightsSetup(location, color?)
Type | Parameter | Default | Description |
---|---|---|---|
Vector | location | Required parameter | No description provided |
Color | color? | Color(1, 0.86, 0.5) | No description provided |

SetSteeringSetup
Configures the Vehicle Steering
my_vehiclewheeled:SetSteeringSetup(steering_type, angle_ratio?)