📁 File
A File represents an entry to a system file.
It is not possible to open files from outside the server folder. All path must be relative to the Server's executable folder. All files are opened as binary file by default.
🎒 Examples
local configuration_file = File("my_awesome_configuration.json")
local configuration_file_json = JSON.parse(configuration_file:Read())
📂 File Access & Sandboxing
Access to files is sandboxed. Only certain directories and files are allowed to be written or read from.
Server Side
On Server side, it's only allowed to access files inside the server folder itself (where server executable is located). Also, accessing the Config.toml is not allowed.
The default directory is where the server executable is located.
Example accessing a file from a Package: Packages/My-Package/Server/MyFile.json
.
Client Side
On the client side, we have the concept of the .transient/
folder which is a folder automatically generated inside the client's cached Packages/
folder, where files can be created in a more persistent way.
It's not allowed to access files outside the client's cache folder Packages/
, and write access is only permitted inside the .transient/
folder itself.
The default directory is in the client's cached Packages/.transient/
folder.
Example accessing a file from a Package (with ready-only access): ../my-package/Client/MyFile.json
.
Example accessing a transient file (with write access): MyFile.json
.
🛠 Constructors
Default Constructor
No description provided
local my_file = File(file_path, truncate?)
Type | Name | Default | Description |
---|---|---|---|
string | file_path | Required parameter | If on Server, this is the Path relative to server executable. Otherwise if on Client, this is the Path relative to Client's 'Packages/.transient/' folder |
boolean | truncate | false | Whether or not to clear the file upon opening it |
🗿 Static Functions
Returns | Name | Description | |
---|---|---|---|
![]() | boolean | CreateDirectory | Creates a Directory (for every folder passed) |
![]() | boolean | Exists | Verifies if a entry exists in the file system |
![]() | table of string | GetDirectories | Gets a list of all directories given a path |
![]() | table of string | GetFiles | Gets a list of all files in a directory |
![]() | string | GetFullPath | Gets the full path given a relative path based on the current side (client or server) |
![]() | boolean | IsDirectory | Checks if a path is a directory |
![]() | boolean | IsRegularFile | Checks if a path is a file |
![]() | integer | Remove | Deletes a folder or file |
![]() | integer | Time | Returns when a file was last modified in Unix time |
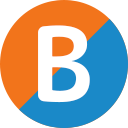
CreateDirectory
Creates a Directory (for every folder passed)
— Returns boolean (if succeeded).
local ret = File.CreateDirectory(path)
Type | Parameter | Default | Description |
---|---|---|---|
string | path | Required parameter | Path to folder |
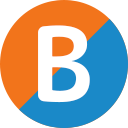
Exists
Verifies if a entry exists in the file system
— Returns boolean (if exists).
local ret = File.Exists(path)
Type | Parameter | Default | Description |
---|---|---|---|
string | path | Required parameter | Path to file or folder |
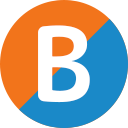
GetDirectories
Gets a list of all directories given a path, optionally with filters
— Returns table of string (List of directories).
local ret = File.GetDirectories(path_filter?, max_depth?)
Type | Parameter | Default | Description |
---|---|---|---|
string | path_filter? |
| Path filter |
integer | max_depth? | -1 | The maximum depth to go further in the folders while searching. Pass -1 for maximum depth |
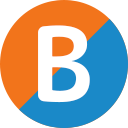
GetFiles
Gets a list of all files in a directory, optionally with filters
— Returns table of string (List of files).
local ret = File.GetFiles(path_filter?, extension_filter?, max_depth?)
Type | Parameter | Default | Description |
---|---|---|---|
string or table | path_filter? |
| Path filter |
string | extension_filter? |
| E.g.: .lua |
integer | max_depth? | -1 | The maximum depth to go further in the folders while searching. Pass -1 for maximum depth |
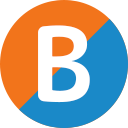
GetFullPath
Gets the full path given a relative path based on the current side (client or server)
— Returns string (the full path parsed).
local ret = File.GetFullPath(path)
Type | Parameter | Default | Description |
---|---|---|---|
string | path | Required parameter | Path to file or directory |
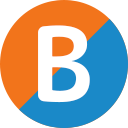
IsDirectory
Checks if a path is a directory
— Returns boolean (if is a directory).
local ret = File.IsDirectory(path)
Type | Parameter | Default | Description |
---|---|---|---|
string | path | Required parameter | Path to folder |
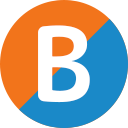
IsRegularFile
Checks if a path is a file
— Returns boolean (if is a regular file).
local ret = File.IsRegularFile(path)
Type | Parameter | Default | Description |
---|---|---|---|
string | path | Required parameter | Path to file |
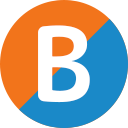
Remove
Deletes a folder or file
— Returns integer (amount of files deleted).
local ret = File.Remove(path)
Type | Parameter | Default | Description |
---|---|---|---|
string | path | Required parameter | Path to file or folder |
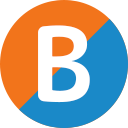
Time
Returns when a file was last modified in Unix time
— Returns integer (the last update time in unix time).
local ret = File.Time(path)
Type | Parameter | Default | Description |
---|---|---|---|
string | path | Required parameter | Path to file |
🦠 Functions
Returns | Name | Description | |
---|---|---|---|
![]() | Close | Closes the file and destroys the entity | |
![]() | Flush | Flushes content to the file | |
![]() | boolean | HasFailed | Checks if the last operation has Failed |
![]() | boolean | IsBad | Checks if the file status is Bad |
![]() | boolean | IsEOF | Checks if the file status is End of File |
![]() | boolean | IsGood | Checks if the file status is Good |
![]() | string | Read | Reads characters from the File and returns it. Also moves the file pointer to the latest read position. Pass 0 to read the whole file |
![]() | ReadAsync | Reads characters from the File asynchronously. | |
![]() | table | ReadJSON | Reads the whole file as a JSON and returns it. |
![]() | ReadJSONAsync | Reads the whole file as a JSON and returns it asynchronously. | |
![]() | string | ReadLine | Reads and returns the next file line |
![]() | Seek | Sets the file pointer to a specific position | |
![]() | integer | Size | Returns the size of the file |
![]() | Skip | Skips n (amount) positions from the current file pointer position | |
![]() | integer | Tell | Returns the current file pointer position |
![]() | Write | Writes the Data at the current position of the file |
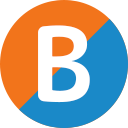
Close
Closes the file and destroys the entity
my_file:Close()
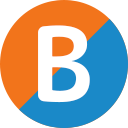
Flush
Flushes content to the file
my_file:Flush()
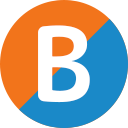
HasFailed
Checks if the last operation has Failed
— Returns boolean (if last operation failed).
local ret = my_file:HasFailed()
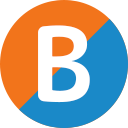
IsBad
Checks if the file status is Bad
— Returns boolean (if status is Bad).
local ret = my_file:IsBad()
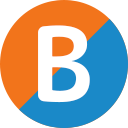
IsEOF
Checks if the file status is End of File
— Returns boolean (if is EOF).
local ret = my_file:IsEOF()
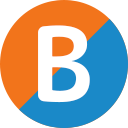
IsGood
Checks if the file status is Good
— Returns boolean (if status is Good).
local ret = my_file:IsGood()
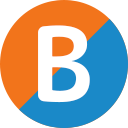
Read
Reads characters from the File and returns it. Also moves the file pointer to the latest read position. Pass 0 to read the whole file
— Returns string (file data).
local ret = my_file:Read(length?)
Type | Parameter | Default | Description |
---|---|---|---|
integer | length? | 0 | Length to be read from file, leave it empty to read the whole file |
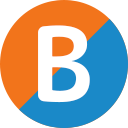
ReadAsync
Reads characters from the File asynchronously.
my_file:ReadAsync(length, callback)
Type | Parameter | Default | Description |
---|---|---|---|
integer | length | Required parameter | Length to be read from file, leave it empty to read the whole file |
function | callback | Required parameter | Callback with this format |
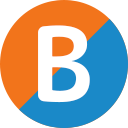
ReadJSON
Reads the whole file as a JSON and returns it.
— Returns table (parsed table).
local ret = my_file:ReadJSON()
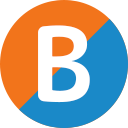
ReadJSONAsync
Reads the whole file as a JSON and returns it asynchronously.
my_file:ReadJSONAsync(callback)
Type | Parameter | Default | Description |
---|---|---|---|
function | callback | Required parameter | Callback with the file read with this format |
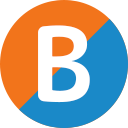
ReadLine
Reads and returns the next file line
— Returns string (file line data).
local ret = my_file:ReadLine()
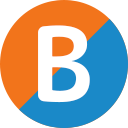
Seek
Sets the file pointer to a specific position
my_file:Seek(position)
Type | Parameter | Default | Description |
---|---|---|---|
integer | position | Required parameter | Position to offset the file pointer |
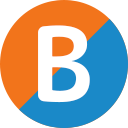
Size
Returns the size of the file
— Returns integer (file size).
local ret = my_file:Size()
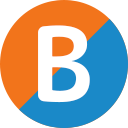
Skip
Skips n (amount) positions from the current file pointer position
my_file:Skip(amount)
Type | Parameter | Default | Description |
---|---|---|---|
integer | amount | Required parameter | Amount to offset the file pointer |
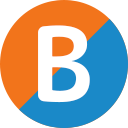
Tell
Returns the current file pointer position
— Returns integer (current file pointer position).
local ret = my_file:Tell()
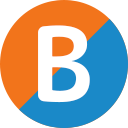
Write
Writes the Data at the current position of the file
my_file:Write(data)
Type | Parameter | Default | Description |
---|---|---|---|
string | data | Required parameter | Data to write to the file |
🚀 Events
This class doesn't have own events.