Base Damageable
Base class for all Damageable entities. It provides Health and Damage related methods and events.
🧑💻API Source
This page is auto-generated! The Functions, Properties and Events described here are defined in our GitHub's API Repository! Feel free to commit suggestions and changes to the source .json API files!
👪Base Class
This is a Base Class. Base Classes are abstract definitions used to share common methods and events between related child classes, thus you cannot spawn it directly.
Classes that share methods and events from this Base Class: Character, CharacterSimple, VehicleWheeled, VehicleWater.
Classes that share methods and events from this Base Class: Character, CharacterSimple, VehicleWheeled, VehicleWater.
🦠 Functions
Returns | Name | Description | |
---|---|---|---|
integer | ApplyDamage | Do damage to this entity | |
![]() | integer | GetHealth | Gets the current health |
![]() | integer | GetMaxHealth | Gets the Max Health |
Respawn | Respawns the Entity, fullying it's Health and moving it to it's Initial Location | ||
SetHealth | Sets the Health of this Entity | ||
SetMaxHealth | Sets the MaxHealth of this Entity |

ApplyDamage
Do damage to this entity, will trigger all related events and apply modified damage based on bone. Also will apply impulse if it's a heavy explosion
— Returns integer (the damage applied).
local ret = my_damageable:ApplyDamage(damage, bone_name?, damage_type?, from_direction?, instigator?, causer?)
Type | Parameter | Default | Description |
---|---|---|---|
integer | damage | Required parameter | No description provided |
string | bone_name? |
| No description provided |
DamageType | damage_type? | DamageType.Shot | No description provided |
Vector | from_direction? | Vector(0, 0, 0) | No description provided |
Player | instigator? | nil | The player which caused the damage |
any | causer? | nil | The object which caused the damage |
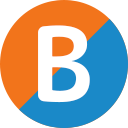
GetHealth
Gets the current health
— Returns integer.
local ret = my_damageable:GetHealth()
See also SetHealth.
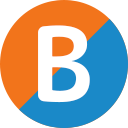
GetMaxHealth
Gets the Max Health
— Returns integer.
local ret = my_damageable:GetMaxHealth()
See also SetMaxHealth.

Respawn
Respawns the Entity, fullying it's Health and moving it to it's Initial Location
my_damageable:Respawn(location?, rotation?)
Type | Parameter | Default | Description |
---|---|---|---|
Vector | location? | initial location | If not passed will use the initial location passed when the Entity spawned |
Rotator | rotation? | Rotator(0, 0, 0) | No description provided |

SetHealth
Sets the Health of this Entity. You can only call it on alive Entities (call Respawn first)
my_damageable:SetHealth(new_health)
Type | Parameter | Default | Description |
---|---|---|---|
integer | new_health | Required parameter | No description provided |
See also GetHealth.

SetMaxHealth
Sets the MaxHealth of this Entity
my_damageable:SetMaxHealth(max_health)
Type | Parameter | Default | Description |
---|---|---|---|
integer | max_health | Required parameter | No description provided |
See also GetMaxHealth.
🚀 Events
Name | Description | |
---|---|---|
![]() | Death | When Entity Dies |
![]() | HealthChange | When Entity has it's Health changed, or because took damage or manually set through scripting or respawning |
![]() | Respawn | When Entity Respawns |
![]() | TakeDamage | Triggered when this Entity takes damage |
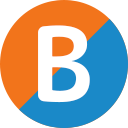
Death
When Entity Dies
Damageable.Subscribe("Death", function(self, last_damage_taken, last_bone_damaged, damage_type_reason, hit_from_direction, instigator, causer)
-- Death was called
end)
Type | Argument | Description |
---|---|---|
Base Damageable | self | No description provided |
integer | last_damage_taken | No description provided |
string | last_bone_damaged | No description provided |
DamageType | damage_type_reason | No description provided |
Vector | hit_from_direction | No description provided |
Player or nil | instigator | No description provided |
Base Actor or nil | causer | The object which caused the damage |
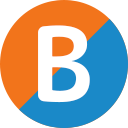
HealthChange
When Entity has it's Health changed, or because took damage or manually set through scripting or respawning
Damageable.Subscribe("HealthChange", function(self, old_health, new_health)
-- HealthChange was called
end)
Type | Argument | Description |
---|---|---|
Base Damageable | self | No description provided |
integer | old_health | No description provided |
integer | new_health | No description provided |
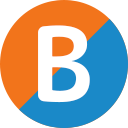
Respawn
When Entity Respawns
Damageable.Subscribe("Respawn", function(self)
-- Respawn was called
end)
Type | Argument | Description |
---|---|---|
Base Damageable | self | No description provided |
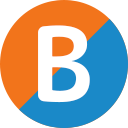
TakeDamage
Triggered when this Entity takes damage
Returnfalse
to cancel the damage (will still display animations, particles and apply impact forces)
Damageable.Subscribe("TakeDamage", function(self, damage, bone, type, from_direction, instigator, causer)
-- TakeDamage was called
end)
Type | Argument | Description |
---|---|---|
Base Damageable | self | No description provided |
integer | damage | No description provided |
string | bone | Damaged bone |
DamageType | type | Damage Type |
Vector | from_direction | Direction of the damage relative to the damaged actor |
Player | instigator | The player which caused the damage |
any | causer | The object which caused the damage |