Base Pawn
Base class for all Character entities.
Classes that share methods and events from this Base Class: Character, CharacterSimple.
🦠 Functions
Returns | Name | Description | |
---|---|---|---|
![]() | AddSkeletalMeshAttached | Attaches a Skeletal Mesh as master pose to this entity | |
![]() | AddStaticMeshAttached | Attaches a Static Mesh to this entity | |
Follow | AI: Makes this Character to follow another actor | ||
![]() | table of string | GetAllSkeletalMeshAttached | Gets all Skeletal Meshes attached to this entity |
![]() | table of string | GetAllStaticMeshAttached | Gets all Static Meshes attached to this entity |
![]() | Rotator | GetControlRotation | Gets the Control Rotation |
![]() | SkeletalMesh Reference | GetMesh | Gets the base Mesh Asset |
![]() | Vector | GetMovingTo | Gets the Moving To location |
![]() | Player or nil | GetPlayer | Gets the possessing Player |
![]() | HideBone | Hides a bone of this Character | |
![]() | boolean | IsBoneHidden | Gets if a bone is hidden |
Jump | Triggers this Character to jump | ||
MoveTo | AI: Makes this Character to walk to the Location | ||
![]() | RemoveAllSkeletalMeshesAttached | Removes all SkeletalMeshes attached | |
![]() | RemoveAllStaticMeshesAttached | Removes all StaticMeshes attached | |
![]() | RemoveSkeletalMeshAttached | Removes, if it exists, a SkeletalMesh from this entity given its custom ID | |
![]() | RemoveStaticMeshAttached | Removes, if it exists, a StaticMesh from this enitity given its custom ID | |
SetBrakingSettings | Sets the Movement Braking Settings of this Character | ||
SetCanCrouch | Sets if this Character is allowed to Crouch and to Prone | ||
SetCanJump | Sets if this Character is allowed to Jump | ||
SetCapsuleSize | Sets this Character's Capsule size | ||
SetJumpZVelocity | Sets the velocity of the jump | ||
![]() | SetStaticMeshAttachedTransform | Sets a Static Mesh Attached location and rotation | |
StopAnimation | Stops an Animation Montage on this character | ||
StopMovement | AI: Stops the movement | ||
![]() | UnHideBone | UnHide a bone of this Character |
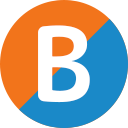
AddSkeletalMeshAttached
Spawns and attaches a SkeletalMesh to this entity, the SkeletalMesh must have the same skeleton used by this Actor's mesh, and will follow all animations from it. Uses a custom ID to be used for removing/customizing it afterwards
my_pawn:AddSkeletalMeshAttached(id, skeletal_mesh_path, use_parent_bounds?)
Type | Parameter | Default | Description |
---|---|---|---|
string | id | Required parameter | Used further for removing or applying material settings on it |
SkeletalMesh Reference | skeletal_mesh_path | Required parameter | Path to SkeletalMesh asset to attach |
boolean | use_parent_bounds? | true | If true, this component uses its parents bounds when attached. This can be a significant optimization with many components attached together |
See also RemoveSkeletalMeshAttached.
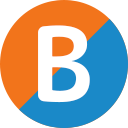
AddStaticMeshAttached
Spawns and attaches a StaticMesh to this entity in a Socket with a relative location and rotation. Uses a custom ID to be used for removing/customizing it afterwards
my_pawn:AddStaticMeshAttached(id, static_mesh_path, socket?, relative_location?, relative_rotation?, use_parent_bounds?)
Type | Parameter | Default | Description |
---|---|---|---|
string | id | Required parameter | Unique ID to assign to the StaticMesh |
StaticMesh Reference | static_mesh_path | Required parameter | Path to StaticMesh asset to attach |
string | socket? |
| Bone socket to attach to |
Vector | relative_location? | Vector(0, 0, 0) | Relative location |
Rotator | relative_rotation? | Rotator(0, 0, 0) | Relative rotation |
boolean | use_parent_bounds? | true | If true, this component uses its parents bounds when attached. This can be a significant optimization with many components attached together |
See also SetStaticMeshAttachedTransform, RemoveStaticMeshAttached.

Follow
AI: Makes this Character to follow another actor
Triggers event MoveComplete
my_pawn:Follow(actor, acceptance_radius?, stop_on_succeed?, stop_on_fail?, update_rate?)
Type | Parameter | Default | Description |
---|---|---|---|
Base Actor | actor | Required parameter | Actor to follow |
float | acceptance_radius? | 50 | Radius to consider success |
boolean | stop_on_succeed? | false | Whether to stop when reaching the target |
boolean | stop_on_fail? | false | Whether to stop when failed to reach the target |
float | update_rate? | 0.25 | How often to recalculate the AI path |
See also StopMovement, MoveTo, GetMovingTo, MoveComplete.
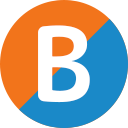
GetAllSkeletalMeshAttached
Gets all Skeletal Meshes attached to this entity
— Returns table of string (the key as the Attached ID, and the value as the Asset Path).
local ret = my_pawn:GetAllSkeletalMeshAttached()
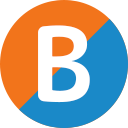
GetAllStaticMeshAttached
Gets all Static Meshes attached to this entity
— Returns table of string (the key as the Attached ID, and the value as the Asset Path).
local ret = my_pawn:GetAllStaticMeshAttached()
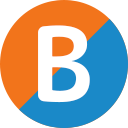
GetControlRotation
Gets the Control Rotation
— Returns Rotator.
local ret = my_pawn:GetControlRotation()
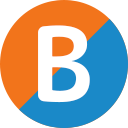
GetMesh
Gets the base Mesh Asset
— Returns SkeletalMesh Reference.
local ret = my_pawn:GetMesh()
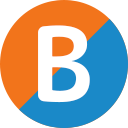
GetMovingTo
Gets the Moving To location
— Returns Vector (the moving to location or Vector(0, 0, 0) if not moving).
local ret = my_pawn:GetMovingTo()
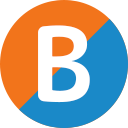
GetPlayer
Gets the possessing Player
local ret = my_pawn:GetPlayer()
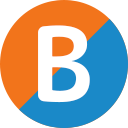
HideBone
Hides a bone of this Character.
Check Bone Names List
my_pawn:HideBone(bone_name?)
Type | Parameter | Default | Description |
---|---|---|---|
string | bone_name? |
| Bone to hide |
See also UnHideBone, IsBoneHidden.
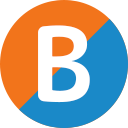
IsBoneHidden
Gets if a bone is hidden
— Returns boolean (if the bone is hidden).
local ret = my_pawn:IsBoneHidden(bone_name)
Type | Parameter | Default | Description |
---|---|---|---|
string | bone_name | Required parameter | Bone to check |
See also UnHideBone, HideBone.

Jump
Triggers this Character to jump
my_pawn:Jump()

MoveTo
AI: Makes this Character to walk to the Location
Triggers event MoveComplete
my_pawn:MoveTo(location, acceptance_radius?)
Type | Parameter | Default | Description |
---|---|---|---|
Vector | location | Required parameter | No description provided |
float | acceptance_radius? | 50 | No description provided |
See also StopMovement, Follow, GetMovingTo, MoveComplete.
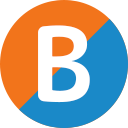
RemoveAllSkeletalMeshesAttached
Removes all SkeletalMeshes attached
my_pawn:RemoveAllSkeletalMeshesAttached()
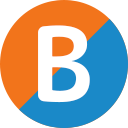
RemoveAllStaticMeshesAttached
Removes all StaticMeshes attached
my_pawn:RemoveAllStaticMeshesAttached()
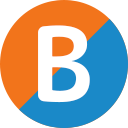
RemoveSkeletalMeshAttached
Removes, if it exists, a SkeletalMesh from this entity given its custom ID
my_pawn:RemoveSkeletalMeshAttached(id)
Type | Parameter | Default | Description |
---|---|---|---|
string | id | Required parameter | Unique ID of the SkeletalMesh to remove |
See also AddSkeletalMeshAttached.
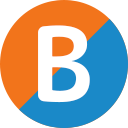
RemoveStaticMeshAttached
Removes, if it exists, a StaticMesh from this enitity given its custom ID
my_pawn:RemoveStaticMeshAttached(id)
Type | Parameter | Default | Description |
---|---|---|---|
string | id | Required parameter | Unique ID of the StaticMesh to remove |
See also AddStaticMeshAttached.

SetBrakingSettings
Sets the Movement Braking Settings of this Character
my_pawn:SetBrakingSettings(ground_friction?, braking_friction_factor?, braking_walking?, braking_flying?, braking_swimming?, braking_falling?)
Type | Parameter | Default | Description |
---|---|---|---|
float | ground_friction? | 2 | No description provided |
float | braking_friction_factor? | 2 | No description provided |
integer | braking_walking? | 96 | No description provided |
integer | braking_flying? | 3000 | No description provided |
integer | braking_swimming? | 10 | No description provided |
integer | braking_falling? | 0 | No description provided |

SetCanCrouch
Sets if this Character is allowed to Crouch and to Prone
my_pawn:SetCanCrouch(can_crouch)
Type | Parameter | Default | Description |
---|---|---|---|
boolean | can_crouch | Required parameter | No description provided |

SetCanJump
Sets if this Character is allowed to Jump
my_pawn:SetCanJump(can_jump)
Type | Parameter | Default | Description |
---|---|---|---|
boolean | can_jump | Required parameter | No description provided |

SetCapsuleSize
Sets this Character's Capsule size (will affect Camera location and Character's collision)
my_pawn:SetCapsuleSize(radius, half_height)
Type | Parameter | Default | Description |
---|---|---|---|
integer | radius | Required parameter | Default is 42 |
integer | half_height | Required parameter | Default is 96 |

SetJumpZVelocity
Sets the velocity of the jump
my_pawn:SetJumpZVelocity(jump_z_velocity)
Type | Parameter | Default | Description |
---|---|---|---|
integer | jump_z_velocity | Required parameter | Default is 450 |
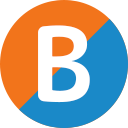
SetStaticMeshAttachedTransform
Sets a Static Mesh Attached location and rotation
my_pawn:SetStaticMeshAttachedTransform(id, relative_location, relative_rotation)
Type | Parameter | Default | Description |
---|---|---|---|
string | id | Required parameter | Unique ID of the StaticMesh set with AddStaticMeshAttached |
Vector | relative_location | Required parameter | New relative location |
Rotator | relative_rotation | Required parameter | New relative rotation |
See also AddStaticMeshAttached.

StopAnimation
Stops an Animation Montage on this character
my_pawn:StopAnimation(animation_asset)
Type | Parameter | Default | Description |
---|---|---|---|
Animation Reference | animation_asset | Required parameter | No description provided |

StopMovement
AI: Stops the movement
Triggers event MoveComplete
my_pawn:StopMovement()
See also Follow, MoveTo, GetMovingTo, MoveComplete.
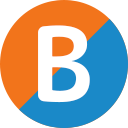
UnHideBone
UnHide a bone of this Character.
Check Bone Names List
my_pawn:UnHideBone(bone_name)
Type | Parameter | Default | Description |
---|---|---|---|
string | bone_name | Required parameter | Bone to unhide |
See also HideBone, IsBoneHidden.
🚀 Events
Name | Description | |
---|---|---|
![]() | MoveComplete | Called when AI reaches it's destination, or when it fails |
![]() | Possess | When Character is possessed |
![]() | UnPossess | When Character is unpossessed |
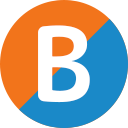
MoveComplete
Called when AI reaches it's destination, or when it fails
Pawn.Subscribe("MoveComplete", function(self, succeeded)
-- MoveComplete was called
end)
See also StopMovement, MoveTo, Follow, GetMovingTo.
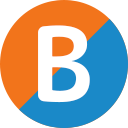
Possess
When Character is possessed
Pawn.Subscribe("Possess", function(self, possesser)
-- Possess was called
end)
See also UnPossess, GetPlayer.
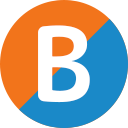
UnPossess
When Character is unpossessed
Pawn.Subscribe("UnPossess", function(self, old_possesser)
-- UnPossess was called
end)