🖥️ WebUI
Class for spawning a dynamic Web Browser.
Our WebUI implementation is built using the last versions of Chromium Embedded Framework.
To access the in-browser remote debugging head over to http://localhost:9222 when you are connected to a server.
Proprietary Codecs like MP3 and AAC are not supported on public CEF builds. We recommend converting your media files to WEBM or OGG.
🎒 Examples
-- Loading a local file
local my_ui = WebUI(
"Awesome UI", -- Name
"file://UI/index.html", -- Path relative to this package (Client/)
WebUIVisibility.Visible -- Is Visible on Screen
)
-- Loading a Web URL
local my_browser = WebUI(
"Awesome Site", -- Name
"https://nanos.world", -- Web's URL
WebUIVisibility.Visible -- Is Visible on Screen
)
-- Loading a local file from other package
local my_ui = WebUI(
"Awesome Other UI", -- Name
"file://other-package/Client/UI/index.html",
WebUIVisibility.Visible -- Is Visible on Screen
)
Using a WebUI as Mesh Material
-- Spawns a WebUI with is_visible = false, is_transparent = false, auto_resize = false and size of 500x500
local my_ui = WebUI("Awesome Site", "https://nanos.world", false, false, false, 500, 500)
-- Spawns a StaticMesh (can be any mesh)
local static_mesh = StaticMesh(Vector(0, 0, 100), Rotator(), "nanos-world::SM_Cube")
-- Sets the mesh material to use the WebUI
static_mesh:SetMaterialFromWebUI(my_ui)
Communicating between Lua and JS (WebUI)
local my_ui = WebUI("Awesome UI", "file://UI/index.html")
local param1 = 123
local param2 = "hello"
-- Calls a JS event
my_ui:CallEvent("MyEvent", param1, param2)
-- Subscribes to receive JS events
my_ui:Subscribe("MyAnswer", function(param1)
Console.Log("Received back! %s", param1)
-- Will output 'Received back! Hey there!'
end)
// Register for "MyEvent" from Lua
Events.Subscribe("MyEvent", function(param1, param2) {
console.log("Triggered! " + param1 + " " + param2);
// Will output 'Triggered! 123 hello'
// Triggers "MyAnswer" on Lua
Events.Call("MyAnswer", "Hey there!");
});
// It is also possible to unsubscribe from an event to make it stop triggering
Events.Unsubscribe("MyEvent");
Pretty Scroll Bar
Since we migrated from Webkit to CEF, some scrollbars got ugly. Here's a small CSS snippet to make them almost like the Webkit ones:
::-webkit-scrollbar {
width: 6px;
}
::-webkit-scrollbar-thumb {
border-radius: 10px;
background-color: #494949;
}
body {
scrollbar-gutter: stable both-edges;
}
More related examples:
User Interfacecore-concepts/scripting/user-interface HUD básica (HTML)getting-started/tutorials-and-examples/basic-hud-htmlYou can use the output Texture from a Canvas with :SetMaterialFromWebUI() method!
📚 Libraries & Frameworks
Here a list of Community Created Libraries & Frameworks making use of WebUIs expanding it's possibilities:
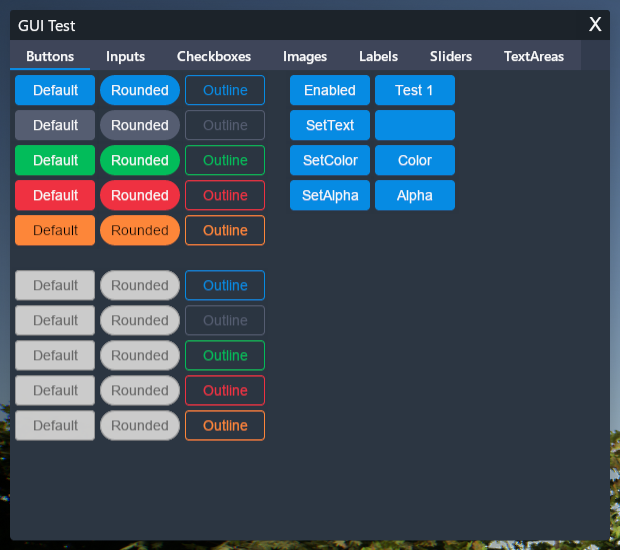
EGUI
Framework for creating User Interfaces by MegaThorx
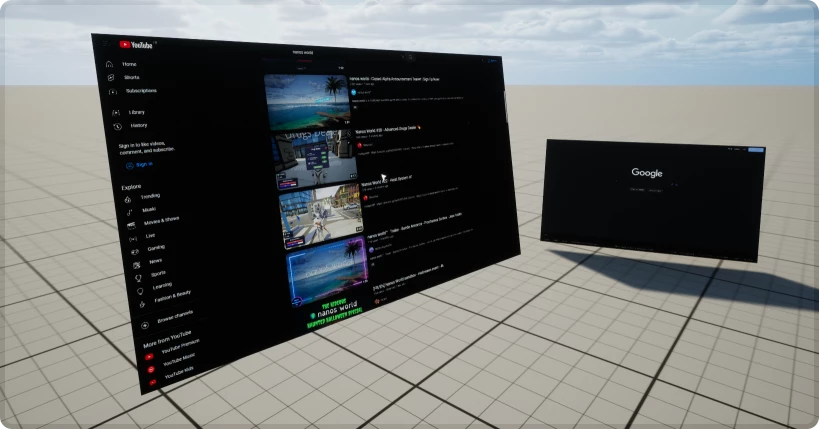
WebUI3d2d
Spawn interactable WebUIs in the 3D world by Timmy

UI Framework
A powerful UI framework ported by Voltaism
🛠 Constructors
Default Constructor
local my_webui = WebUI(name, path, visibility?, is_transparent?, auto_resize?, width?, height?)
Type | Name | Default | Description |
---|---|---|---|
string | name | Required parameter | Used for debugging logs |
HTML Path | path | Required parameter | Web URL or HTML File Path as file://my_file.html |
WidgetVisibility | visibility | WidgetVisibility.Visible | if WebUI is visible on screen |
boolean | is_transparent | true | if WebUI background is transparent |
boolean | auto_resize | true | if should auto resize when screen changes it's size (useful OFF when you are painting meshes with WebUI) |
integer | width | 0 | size of the WebUI width when you are not using auto_resize |
integer | height | 0 | size of the WebUI height when you are not using auto_resize |
🔍 HTML Path Searchers
Loading a .html file supports the following searchers, which are looked in the following order:
- Relative to
current-file-path/
- Relative to
current-package/Client/
- Relative to
current-package/
- Relative to
Packages/