🔣 Blueprint
A Blueprint Class allows spawning any Unreal Blueprint Actor in nanos world.
If your Actor Blueprint was spawned on the Server, it will be automatically synchronized with other players using the nanos world Network Authority system! It follows the same rules as all other entities!
🎒 Examples
Calling Blueprint Events from lua
-- Spawns the Blueprint
local blueprint = Blueprint(Vector(), Rotator(), "my-asset-pack::BP_MyBlueprint")
local param1 = 123
local param2 = "hello there!"
-- Calls the event, passing any parameters
blueprint:CallBlueprintEvent("MyBlueprintCustomEvent", param1, param2)
Binding Blueprint Event Dispatchers
-- Spawns the Blueprint
local blueprint = Blueprint(Vector(), Rotator(), "my-asset-pack::BP_MyBlueprint")
-- Subscribes to a Blueprint Event Dispatcher
blueprint:BindBlueprintEventDispatcher("MyBlueprintDispatcher", function(self, arg1, arg2)
Console.Log("Called from Blueprint!", arg1, arg2)
end)
🛠 Constructors
Default Constructor
local my_blueprint = Blueprint(location, rotation, blueprint_asset, collision_type?)
Type | Name | Default | Description |
---|---|---|---|
Vector | location | Required parameter | No description provided |
Rotator | rotation | Required parameter | No description provided |
Blueprint Reference | blueprint_asset | Required parameter | No description provided |
CollisionType | collision_type | CollisionType.Auto | No description provided |
🗿 Static Functions
Inherited Entity Static Functions
🦠 Functions
Inherited Entity Functions
Inherited Actor Functions
Inherited Paintable Functions
Returns | Name | Description | |
---|---|---|---|
function | BindBlueprintEventDispatcher | Assigns and Binds a Blueprint Event Dispatcher | |
![]() | varargs of any | CallBlueprintEvent | Calls a Blueprint Event or Function |
any | GetBlueprintPropertyValue | Gets a Blueprint Property/Variable value | |
SetBlueprintPropertyValue | Sets a Blueprint Property/Variable value directly | ||
UnbindBlueprintEventDispatcher | Unbinds a Blueprint Event Dispatcher |

BindBlueprintEventDispatcher
Assigns and Binds a Blueprint Event Dispatcher
— Returns function (the callback itself).
local ret = my_blueprint:BindBlueprintEventDispatcher(dispatcher_name, callback)
Type | Parameter | Default | Description |
---|---|---|---|
string | dispatcher_name | Required parameter | Event Dispatcher name |
function | callback | Required parameter | Callback function to call with this format |
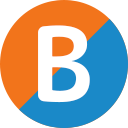
CallBlueprintEvent
Calls a Blueprint Event or Function
Returns all Function return values on Client Side
— Returns varargs of any (the function return values).
local ret_01, ret_02, ... = my_blueprint:CallBlueprintEvent(event_name, arguments...?)
Type | Parameter | Default | Description |
---|---|---|---|
string | event_name | Required parameter | Event or Function name |
any | arguments...? | nil | Sequence of arguments to pass to the event |

GetBlueprintPropertyValue
Gets a Blueprint Property/Variable value
— Returns any (the value).
local ret = my_blueprint:GetBlueprintPropertyValue(property_name)
Type | Parameter | Default | Description |
---|---|---|---|
string | property_name | Required parameter | No description provided |
See also SetBlueprintPropertyValue.

SetBlueprintPropertyValue
Sets a Blueprint Property/Variable value directly
my_blueprint:SetBlueprintPropertyValue(property_name, value)
Type | Parameter | Default | Description |
---|---|---|---|
string | property_name | Required parameter | No description provided |
any | value | Required parameter | No description provided |
See also GetBlueprintPropertyValue.

UnbindBlueprintEventDispatcher
Unbinds a Blueprint Event Dispatcher
my_blueprint:UnbindBlueprintEventDispatcher(dispatcher_name, callback?)
Type | Parameter | Default | Description |
---|---|---|---|
string | dispatcher_name | Required parameter | Event Dispatcher name |
function | callback? | Required parameter | Optional callback to unbind |
🚀 Events
Inherited Entity Events
Inherited Actor Events
✅ List of Supported Parameter Types
List of all supported parameters which can be passed between Lua ↔ Blueprint:
Lua Type | Blueprint Type | Lua → BP | BP → Lua |
---|---|---|---|
boolean | Boolean | ✅ | ✅ |
number | Byte | ✅ | ✅ |
number | Integer | ✅ | ✅ |
number | Integer64 | ✅ | ✅ |
number | Float | ✅ | ✅ |
number | Enum | ✅ | ✅ |
string | String | ✅ | ✅ |
string | Name | ✅ | ✅ |
string | Text | ✅ | ✅ |
Vector2D | Vector2D | ✅ | ✅ |
Vector | Vector | ✅ | ✅ |
Rotator | Rotator | ✅ | ✅ |
Color | Color | ✅ | ✅ |
Color | LinearColor | ✅ | ✅ |
StaticMesh | StaticMeshActor | ✅ | ✅ |
Prop | StaticMeshActor | ✅ | ✅ |
Weapon | SkeletalMeshActor | ✅ | ✅ |
Vehicle | WheeledVehiclePawn | ✅ | ✅ |
Grenade | StaticMeshActor | ✅ | ✅ |
Melee | StaticMeshActor | ✅ | ✅ |
Light | Light | ✅ | ✅ |
Decal | DecalActor | ✅ | ✅ |
TextRender | WorldText3D | ✅ | ✅ |
Sound | AmbientSound | ✅ | ✅ |
Canvas | MaterialInstanceDynamic | ✅ | ❌ |
WebUI | MaterialInstanceDynamic | ✅ | ❌ |
SceneCapture | MaterialInstanceDynamic | ✅ | ❌ |
Blueprint | Actor | ✅ | ✅ |
Widget | Widget | ✅ | ❌ |
BaseActor | Actor | ✅ | ✅ |
Player | PlayerController | ✅ | ❌ |
SpecialPath | Texture2D | ✅ | ❌ |
string | Class | ✅ | ❌ |
table | Transform | ✅ | ✅ |
table | SlateBrush | ✅ | ✅ |
table | SlateFontInfo | ✅ | ✅ |
table | Any Struct | ✅ | ✅ |
It is only possible to pass Actors from Blueprint → Lua if the Actor is a Spawned Entity. It is not possible to pass newly spawned Actors in Blueprints to Lua.
Passing Maps
, Arrays
and Sets
is also supported! As long their keys/values are in the list above.
Any Struct
Custom Structs can also be passed and retrieved, just pass an object with the same properties as the Unreal Struct, examples:
FTransform
{
["Translation"] = Vector(),
["Rotation"] = Rotator(),
["Scale"] = Vector()
}
FSlateBrush
{
["DrawAs"] = 0, -- ESlateBrushDrawType
["Tiling"] = 0, -- ESlateBrushTileType
["Mirroring"] = 0, -- ESlateBrushMirrorType
["ImageSize"] = Vector2D(),
["Tint"] = Color(),
["Image"] = "package://sandbox/Client/my_image.jpg", -- Special Path, or Canvas, WebUI, SceneCapture
["Margin"] = {}, -- FMargin
["OutlineSettings"] = {} -- FSlateBrushOutlineSettings
}
FSlateFontInfo
{
["FontFamily"] = "package://my-package/Client/my_font.ttf", -- Special Path
["FontMaterial"] = "my-asset-pack::M_MyMaterial", -- Material Path
["OutlineSettings"] = {} -- FSlateBrushOutlineSettings
["Typeface"] = "", -- string
["Size"] = 24,
["LetterSpacing"] = 0,
["SkewAmount"] = 0.0
}
FMargin
{
["Bottom"] = 0.0,
["Left"] = 0.0,
["Right"] = 0.0,
["Top"] = 0.0
}
FVector4
{
["W"] = 0.0,
["X"] = 0.0,
["Y"] = 0.0,
["Z"] = 0.0
}