🔣 Blueprint
A Blueprint Class allows spawning any Unreal Blueprint Actor in nanos world.
Si votre acteur Blueprint a été créé sur le Serveur, il sera automatiquement synchronisé avec les autres joueurs en utilisant le système d'Autorité de Réseau de nanos world ! Il suit les mêmes règles que toutes les autres entités !
🎒 Examples
Calling Blueprint Events from lua
-- Spawns the Blueprint
local blueprint = Blueprint(Vector(), Rotator(), "my-asset-pack::BP_MyBlueprint")
local param1 = 123
local param2 = "hello there!"
-- Calls the event, passing any parameters
blueprint:CallBlueprintEvent("MyBlueprintCustomEvent", param1, param2)
Binding Blueprint Event Dispatchers
-- Spawns the Blueprint
local blueprint = Blueprint(Vector(), Rotator(), "my-asset-pack::BP_MyBlueprint")
-- Subscribes to a Blueprint Event Dispatcher
blueprint:BindBlueprintEventDispatcher("MyBlueprintDispatcher", function(self, arg1, arg2)
Console.Log("Called from Blueprint!", arg1, arg2)
end)
🛠 Constructors
Default Constructor
local my_blueprint = Blueprint(location, rotation, blueprint_asset, collision_type?)
Type | Name | Default | Description |
---|---|---|---|
Vector | location | Required parameter | No description provided |
Rotator | rotation | Required parameter | No description provided |
Blueprint Reference | blueprint_asset | Required parameter | No description provided |
CollisionType | collision_type | CollisionType.Auto | No description provided |
🗿 Static Functions
Inherited Entity Static Functions
Base Entityscripting-reference/classes/base-classes/Entity
Returns | Name | Description | |
---|---|---|---|
![]() | table of Base Entity | GetAll | Returns a table containing all Entities of the class this is called on |
![]() | Base Entity | GetByIndex | Returns a specific Entity of this class at an index |
![]() | integer | GetCount | Returns how many Entities of this class exist |
![]() | table of table | GetInheritedClasses | Gets a list of all directly inherited classes from this Class created with the Inheriting System |
![]() | iterator | GetPairs | Returns an iterator with all Entities of this class to be used with pairs() |
![]() | table or nil | GetParentClass | Gets the parent class if this Class was created with the Inheriting System |
![]() | table | Inherit | Inherits this class with the Inheriting System |
![]() | boolean | IsChildOf | Gets if this Class is child of another class if this Class was created with the Inheriting System |
![]() | function | Subscribe | Subscribes to an Event for all entities of this Class |
![]() | function | SubscribeRemote | Subscribes to a custom event called from server |
![]() | Unsubscribe | Unsubscribes all callbacks from this Event in this Class within this Package, or only the callback passed |
🦠 Functions
Inherited Entity Functions
Base Entityscripting-reference/classes/base-classes/Entity
Returns | Name | Description | |
---|---|---|---|
BroadcastRemoteEvent | Calls a custom remote event directly on this entity to all Players | ||
CallRemoteEvent | Calls a custom remote event directly on this entity to a specific Player | ||
CallRemoteEvent | Calls a custom remote event directly on this entity | ||
Destroy | Destroys this Entity | ||
table of string | GetAllValuesKeys | Gets a list of all values keys | |
![]() | table | GetClass | Gets the class of this entity |
![]() | integer | GetID | Gets the universal network ID of this Entity (same on both client and server) |
![]() | any | GetValue | Gets a Value stored on this Entity at the given key |
![]() | boolean | IsA | Recursively checks if this entity is inherited from a Class |
![]() | boolean | IsValid | Returns true if this Entity is valid (i.e. wasn't destroyed and points to a valid Entity) |
![]() | SetValue | Sets a Value in this Entity | |
![]() | function | Subscribe | Subscribes to an Event on this specific entity |
![]() | function | SubscribeRemote | Subscribes to a custom event called from server on this specific entity |
![]() | Unsubscribe | Unsubscribes all callbacks from this Event in this Entity within this Package, or only the callback passed |
Inherited Actor Functions
Base Actorscripting-reference/classes/base-classes/Actor
Returns | Name | Description | |
---|---|---|---|
AddActorTag | Adds an Unreal Actor Tag to this Actor | ||
![]() | AddImpulse | Applies a force in world world to this Actor | |
AttachTo | Attaches this Actor to any other Actor, optionally at a specific bone | ||
Detach | Detaches this Actor from AttachedTo Actor | ||
table of string | GetActorTags | Gets all Unreal Actor Tags on this Actor | |
![]() | table of Base Actor | GetAttachedEntities | Gets all Actors attached to this Actor |
![]() | Base Actor or nil | GetAttachedTo | Gets the Actor this Actor is attached to |
table | GetBounds | Gets this Actor's bounds | |
![]() | CollisionType | GetCollision | Gets this Actor's collision type |
![]() | integer | GetDimension | Gets this Actor's dimension |
float | GetDistanceFromCamera | Gets the distance of this Actor from the Camera | |
![]() | Vector | GetForce | Gets this Actor's force (set by SetForce() ) |
![]() | Vector | GetLocation | Gets this Actor's location in the game world |
Player or nil | GetNetworkAuthority | Gets this Actor's Network Authority Player | |
![]() | Vector | GetRelativeLocation | Gets this Actor's Relative Location if it's attached |
![]() | Rotator | GetRelativeRotation | Gets this Actor's Relative Rotation if it's attached |
![]() | Rotator | GetRotation | Gets this Actor's angle in the game world |
![]() | Vector | GetScale | Gets this Actor's scale |
float | GetScreenPercentage | Gets the percentage of this Actor size in the screen | |
![]() | Vector | GetVelocity | Gets this Actor's current velocity |
boolean | HasAuthority | Gets if this Actor was spawned by the client side | |
boolean | HasNetworkAuthority | Returns true if the local Player is currently the Network Authority of this Actor | |
![]() | boolean | IsBeingDestroyed | Returns true if this Actor is being destroyed |
![]() | boolean | IsGravityEnabled | Returns true if gravity is enabled on this Actor |
![]() | boolean | IsInWater | Returns true if this Actor is in water |
![]() | boolean | IsNetworkDistributed | Returns true if this Actor is currently network distributed |
![]() | boolean | IsVisible | Returns true if this Actor is visible |
RemoveActorTag | Removes an Unreal Actor Tag from this Actor | ||
![]() | RotateTo | Smoothly rotates this actor to an angle over a certain time | |
SetCollision | Sets this Actor's collision type | ||
SetDimension | Sets this Actor's Dimension | ||
![]() | SetForce | Adds a permanent force to this Actor, set to Vector(0, 0, 0) to cancel | |
SetGravityEnabled | Sets whether gravity is enabled on this Actor | ||
SetHighlightEnabled | Sets whether the highlight is enabled on this Actor, and which highlight index to use | ||
SetLifeSpan | Sets the time (in seconds) before this Actor is destroyed. After this time has passed, the actor will be automatically destroyed. | ||
SetLocation | Sets this Actor's location in the game world | ||
SetNetworkAuthority | Sets the Player to have network authority over this Actor | ||
SetNetworkAuthorityAutoDistributed | Sets if this Actor will auto distribute the network authority between players | ||
SetOutlineEnabled | Sets whether the outline is enabled on this Actor, and which outline index to use | ||
SetRelativeLocation | Sets this Actor's relative location in local space (only if this actor is attached) | ||
SetRelativeRotation | Sets this Actor's relative rotation in local space (only if this actor is attached) | ||
SetRotation | Sets this Actor's rotation in the game world | ||
SetScale | Sets this Actor's scale | ||
![]() | SetVisibility | Sets whether the actor is visible or not | |
![]() | TranslateTo | Smoothly moves this actor to a location over a certain time | |
boolean | WasRecentlyRendered | Gets if this Actor was recently rendered on screen |
Inherited Paintable Functions
Base Paintablescripting-reference/classes/base-classes/Paintable
Returns | Name | Description | |
---|---|---|---|
![]() | ResetMaterial | Resets the material from the specified index to the original one | |
![]() | SetMaterial | Sets the material at the specified index of this Actor | |
![]() | SetMaterialColorParameter | Sets a Color parameter in this Actor's material | |
SetMaterialFromCanvas | Sets the material at the specified index of this Actor to a Canvas object | ||
SetMaterialFromSceneCapture | Sets the material at the specified index of this Actor to a SceneCapture object | ||
SetMaterialFromWebUI | Sets the material at the specified index of this Actor to a WebUI object | ||
![]() | SetMaterialScalarParameter | Sets a Scalar parameter in this Actor's material | |
![]() | SetMaterialTextureParameter | Sets a texture parameter in this Actor's material to an image on disk | |
![]() | SetMaterialVectorParameter | Sets a Vector parameter in this Actor's material | |
![]() | SetPhysicalMaterial | Overrides this Actor's Physical Material with a new one |
Returns | Name | Description | |
---|---|---|---|
function | BindBlueprintEventDispatcher | Assigns and Binds a Blueprint Event Dispatcher | |
![]() | varargs of any | CallBlueprintEvent | Calls a Blueprint Event or Function |
any | GetBlueprintPropertyValue | Gets a Blueprint Property/Variable value | |
SetBlueprintPropertyValue | Sets a Blueprint Property/Variable value directly | ||
UnbindBlueprintEventDispatcher | Unbinds a Blueprint Event Dispatcher |

BindBlueprintEventDispatcher
Assigns and Binds a Blueprint Event Dispatcher
— Returns function (the callback itself).
local ret = my_blueprint:BindBlueprintEventDispatcher(dispatcher_name, callback)
Type | Parameter | Default | Description |
---|---|---|---|
string | dispatcher_name | Required parameter | Event Dispatcher name |
function | callback | Required parameter | Callback function to call with this format |
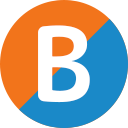
CallBlueprintEvent
Calls a Blueprint Event or Function
Returns all Function return values on Client Side
— Returns varargs of any (the function return values).
local ret_01, ret_02, ... = my_blueprint:CallBlueprintEvent(event_name, arguments...?)
Type | Parameter | Default | Description |
---|---|---|---|
string | event_name | Required parameter | Event or Function name |
any | arguments...? | nil | Sequence of arguments to pass to the event |

GetBlueprintPropertyValue
Gets a Blueprint Property/Variable value
— Returns any (the value).
local ret = my_blueprint:GetBlueprintPropertyValue(property_name)
Type | Parameter | Default | Description |
---|---|---|---|
string | property_name | Required parameter | No description provided |
See also SetBlueprintPropertyValue.

SetBlueprintPropertyValue
Sets a Blueprint Property/Variable value directly
my_blueprint:SetBlueprintPropertyValue(property_name, value)
Type | Parameter | Default | Description |
---|---|---|---|
string | property_name | Required parameter | No description provided |
any | value | Required parameter | No description provided |
See also GetBlueprintPropertyValue.

UnbindBlueprintEventDispatcher
Unbinds a Blueprint Event Dispatcher
my_blueprint:UnbindBlueprintEventDispatcher(dispatcher_name, callback?)
Type | Parameter | Default | Description |
---|---|---|---|
string | dispatcher_name | Required parameter | Event Dispatcher name |
function | callback? | Required parameter | Optional callback to unbind |
🚀 Events
Inherited Entity Events
Base Entityscripting-reference/classes/base-classes/Entity
Name | Description | |
---|---|---|
![]() | ClassRegister | Triggered when a new Class is registered with the Inheriting System |
![]() | Destroy | Triggered when an Entity is destroyed |
![]() | Spawn | Triggered when an Entity is spawned/created |
![]() | ValueChange | Triggered when an Entity has a value changed with :SetValue() |
Inherited Actor Events
Base Actorscripting-reference/classes/base-classes/Actor
Name | Description | |
---|---|---|
DimensionChange | Triggered when an Actor changes it's dimension |
✅ List of Supported Parameter Types
List of all supported parameters which can be passed between Lua ↔ Blueprint:
Lua Type | Blueprint Type | Lua → BP | BP → Lua |
---|---|---|---|
boolean | Boolean | ✅ | ✅ |
number | Byte | ✅ | ✅ |
number | Integer | ✅ | ✅ |
number | Integer64 | ✅ | ✅ |
number | Float | ✅ | ✅ |
number | Enum | ✅ | ✅ |
string | String | ✅ | ✅ |
string | Name | ✅ | ✅ |
string | Text | ✅ | ✅ |
Vector2D | Vector2D | ✅ | ✅ |
Vector | Vector | ✅ | ✅ |
Rotator | Rotator | ✅ | ✅ |
Color | Color | ✅ | ✅ |
Color | LinearColor | ✅ | ✅ |
StaticMesh | StaticMeshActor | ✅ | ✅ |
Prop | StaticMeshActor | ✅ | ✅ |
Weapon | SkeletalMeshActor | ✅ | ✅ |
Vehicle | WheeledVehiclePawn | ✅ | ✅ |
Grenade | StaticMeshActor | ✅ | ✅ |
Melee | StaticMeshActor | ✅ | ✅ |
Light | Light | ✅ | ✅ |
Decal | DecalActor | ✅ | ✅ |
TextRender | WorldText3D | ✅ | ✅ |
Sound | AmbientSound | ✅ | ✅ |
Canvas | MaterialInstanceDynamic | ✅ | ❌ |
WebUI | MaterialInstanceDynamic | ✅ | ❌ |
SceneCapture | MaterialInstanceDynamic | ✅ | ❌ |
Blueprint | Actor | ✅ | ✅ |
Widget | Widget | ✅ | ❌ |
BaseActor | Actor | ✅ | ✅ |
Player | PlayerController | ✅ | ❌ |
SpecialPath | Texture2D | ✅ | ❌ |
string | Class | ✅ | ❌ |
table | Transform | ✅ | ✅ |
table | SlateBrush | ✅ | ✅ |
table | SlateFontInfo | ✅ | ✅ |
table | Any Struct | ✅ | ✅ |
It is only possible to pass Actors from Blueprint → Lua if the Actor is a Spawned Entity. It is not possible to pass newly spawned Actors in Blueprints to Lua.
Passing Maps
, Arrays
and Sets
is also supported! As long their keys/values are in the list above.
Any Struct
Custom Structs can also be passed and retrieved, just pass an object with the same properties as the Unreal Struct, examples:
FTransform
{
["Translation"] = Vector(),
["Rotation"] = Rotator(),
["Scale"] = Vector()
}
FSlateBrush
{
["DrawAs"] = 0, -- ESlateBrushDrawType
["Tiling"] = 0, -- ESlateBrushTileType
["Mirroring"] = 0, -- ESlateBrushMirrorType
["ImageSize"] = Vector2D(),
["Tint"] = Color(),
["Image"] = "package://sandbox/Client/my_image.jpg", -- Special Path, or Canvas, WebUI, SceneCapture
["Margin"] = {}, -- FMargin
["OutlineSettings"] = {} -- FSlateBrushOutlineSettings
}
FSlateFontInfo
{
["FontFamily"] = "package://my-package/Client/my_font.ttf", -- Special Path
["FontMaterial"] = "my-asset-pack::M_MyMaterial", -- Material Path
["OutlineSettings"] = {} -- FSlateBrushOutlineSettings
["Typeface"] = "", -- string
["Size"] = 24,
["LetterSpacing"] = 0,
["SkewAmount"] = 0.0
}
FMargin
{
["Bottom"] = 0.0,
["Left"] = 0.0,
["Right"] = 0.0,
["Top"] = 0.0
}
FVector4
{
["W"] = 0.0,
["X"] = 0.0,
["Y"] = 0.0,
["Z"] = 0.0
}