Base Vehicle
Base class for all Vehicle entities.
🧑💻API Source
This page is auto-generated! The Functions, Properties and Events described here are defined in our GitHub's API Repository! Feel free to commit suggestions and changes to the source .json API files!
👪Base Class
This is a Base Class. Base Classes are abstract definitions used to share common methods and events between related child classes, thus you cannot spawn it directly.
Classes that share methods and events from this Base Class: VehicleWheeled, VehicleWater.
Classes that share methods and events from this Base Class: VehicleWheeled, VehicleWater.
🦠 Functions
Returns | Name | Description | |
---|---|---|---|
![]() | AddSkeletalMeshAttached | Attaches a Skeletal Mesh as master pose to this entity | |
![]() | AddStaticMeshAttached | Attaches a Static Mesh to this entity | |
![]() | table of string | GetAllSkeletalMeshAttached | Gets all Skeletal Meshes attached to this entity |
![]() | table of string | GetAllStaticMeshAttached | Gets all Static Meshes attached to this entity |
table | GetBoneTransform | Gets a Bone Transform in world space given a bone name | |
![]() | table | GetDoors | Gets all configured Doors |
![]() | SkeletalMesh Reference | GetMesh | Gets the Asset name |
![]() | Character | GetPassenger | Gets a passenger from a seat |
![]() | table of Character | GetPassengers | Gets all passengers |
![]() | PlayAnimation | Plays an Animation on this Vehicle | |
![]() | RemoveAllSkeletalMeshesAttached | Removes all SkeletalMeshes attached | |
![]() | RemoveAllStaticMeshesAttached | Removes all StaticMeshes attached | |
![]() | RemoveSkeletalMeshAttached | Removes, if it exists, a SkeletalMesh from this Pickable given its custom ID | |
![]() | RemoveStaticMeshAttached | Removes, if it exists, a StaticMesh from this Pickable given its custom ID | |
SetDoor | Adds a Door to the Vehicle | ||
SetExplosionSettings | Configures the Explosion Settings when health reaches zero | ||
![]() | SetStaticMeshAttachedTransform | Sets a Static Mesh Attached location and rotation |
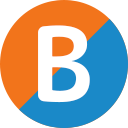
AddSkeletalMeshAttached
Spawns and attaches a SkeletalMesh to this Pickable, the SkeletalMesh must have the same skeleton used by this Actor's mesh, and will follow all animations from it. Uses a custom ID to be used for removing/customizing it afterwards
my_vehicle:AddSkeletalMeshAttached(id, skeletal_mesh_path, use_parent_bounds?)
Type | Parameter | Default | Description |
---|---|---|---|
string | id | Required parameter | Used further for removing or applying material settings on it |
SkeletalMesh Reference | skeletal_mesh_path | Required parameter | Path to SkeletalMesh asset to attach |
boolean | use_parent_bounds? | true | If true, this component uses its parents bounds when attached. This can be a significant optimization with many components attached together |
See also RemoveSkeletalMeshAttached.
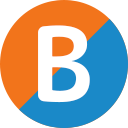
AddStaticMeshAttached
Spawns and attaches a StaticMesh to this Pickable in a Socket with a relative location and rotation. Uses a custom ID to be used for removing/customizing it afterwards
my_vehicle:AddStaticMeshAttached(id, static_mesh_path, socket?, relative_location?, relative_rotation?, use_parent_bounds?)
Type | Parameter | Default | Description |
---|---|---|---|
string | id | Required parameter | Unique ID to assign to the StaticMesh |
StaticMesh Reference | static_mesh_path | Required parameter | Path to StaticMesh asset to attach |
string | socket? |
| Bone socket to attach to |
Vector | relative_location? | Vector(0, 0, 0) | Relative location |
Rotator | relative_rotation? | Rotator(0, 0, 0) | Relative rotation |
boolean | use_parent_bounds? | true | If true, this component uses its parents bounds when attached. This can be a significant optimization with many components attached together |
See also SetStaticMeshAttachedTransform, RemoveStaticMeshAttached.
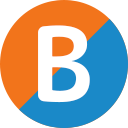
GetAllSkeletalMeshAttached
Gets all Skeletal Meshes attached to this entity
— Returns table of string (the key as the Attached ID, and the value as the Asset Path).
local ret = my_vehicle:GetAllSkeletalMeshAttached()
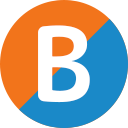
GetAllStaticMeshAttached
Gets all Static Meshes attached to this entity
— Returns table of string (the key as the Attached ID, and the value as the Asset Path).
local ret = my_vehicle:GetAllStaticMeshAttached()

GetBoneTransform
Gets a Bone Transform in world space given a bone name
— Returns table (with this format).
local ret = my_vehicle:GetBoneTransform(bone_name)
Type | Parameter | Default | Description |
---|---|---|---|
string | bone_name | Required parameter | No description provided |
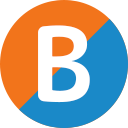
GetDoors
Gets all configured Doors
— Returns table (with this format).
local ret = my_vehicle:GetDoors()
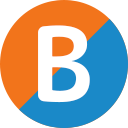
GetMesh
Gets the Asset name
— Returns SkeletalMesh Reference (asset path).
local ret = my_vehicle:GetMesh()
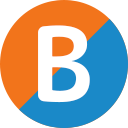
GetPassenger
Gets a passenger from a seat
— Returns Character (or nil if no passenger in seat).
local ret = my_vehicle:GetPassenger(seat)
Type | Parameter | Default | Description |
---|---|---|---|
integer | seat | Required parameter | No description provided |
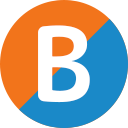
GetPassengers
Gets all passengers
local ret = my_vehicle:GetPassengers()
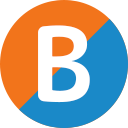
PlayAnimation
Plays an Animation on this Vehicle
my_vehicle:PlayAnimation(animation_path, slot_name?, loop_indefinitely?, blend_in_time?, blend_out_time?, play_rate?, stop_all_montages?)
Type | Parameter | Default | Description |
---|---|---|---|
Animation Reference | animation_path | Required parameter | No description provided |
string | slot_name? | DefaultSlot | No description provided |
boolean | loop_indefinitely? | false | This parameter is only used if the Vehicle has an Animation Blueprint |
float | blend_in_time? | 0.25 | This parameter is only used if the Vehicle has an Animation Blueprint |
float | blend_out_time? | 0.25 | This parameter is only used if the Vehicle has an Animation Blueprint |
float | play_rate? | 1.0 | This parameter is only used if the Vehicle has an Animation Blueprint |
boolean | stop_all_montages? | false | Stops all running Montages from the same Group. This parameter is only used if the Vehicle has an Animation Blueprint |
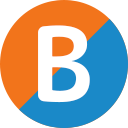
RemoveAllSkeletalMeshesAttached
Removes all SkeletalMeshes attached
my_vehicle:RemoveAllSkeletalMeshesAttached()
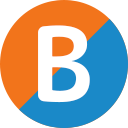
RemoveAllStaticMeshesAttached
Removes all StaticMeshes attached
my_vehicle:RemoveAllStaticMeshesAttached()
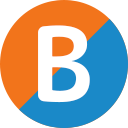
RemoveSkeletalMeshAttached
Removes, if it exists, a SkeletalMesh from this Pickable given its custom ID
my_vehicle:RemoveSkeletalMeshAttached(id)
Type | Parameter | Default | Description |
---|---|---|---|
string | id | Required parameter | Unique ID of the SkeletalMesh to remove |
See also AddSkeletalMeshAttached.
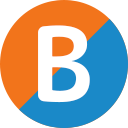
RemoveStaticMeshAttached
Removes, if it exists, a StaticMesh from this Pickable given its custom ID
my_vehicle:RemoveStaticMeshAttached(id)
Type | Parameter | Default | Description |
---|---|---|---|
string | id | Required parameter | Unique ID of the StaticMesh to remove |
See also AddStaticMeshAttached.

SetDoor
Adds a Door at offset_location from root which will pose the Character at seat_location with seat_rotation rotation.
my_vehicle:SetDoor(seat_index, offset_location, seat_location, seat_rotation, trigger_radius, leave_lateral_offset)
Type | Parameter | Default | Description |
---|---|---|---|
integer | seat_index | Required parameter | No description provided |
Vector | offset_location | Required parameter | No description provided |
Vector | seat_location | Required parameter | No description provided |
Rotator | seat_rotation | Required parameter | No description provided |
integer | trigger_radius | Required parameter | No description provided |
integer | leave_lateral_offset | Required parameter | It's where the Character will be ejected when leaving it (e.g. -150 for left door or 150 for right door) |

SetExplosionSettings
Configures the Explosion Settings when health reaches zero
my_vehicle:SetExplosionSettings(engine_relative_location, materials_index_burnt_override, materials_index_burnt_invisible)
Type | Parameter | Default | Description |
---|---|---|---|
Vector | engine_relative_location | Required parameter | Relative location of the Engine. Used to apply particles and effects. |
table | materials_index_burnt_override | Required parameter | List of material indexes to override to burnt when exploded. Leave it empty for all indexes. |
table | materials_index_burnt_invisible | Required parameter | List of material indexes to override to invisible when exploded. Leave it empty for all indexes. |
Vehicle.SetExplosionSettings Examples
Vehicle Explosion Settings
my_vehicle:SetExplosionSettings(Vector(-50, 0, 0), { 1, 2, 4 }, { 3 })
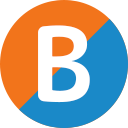
SetStaticMeshAttachedTransform
Sets a Static Mesh Attached location and rotation
my_vehicle:SetStaticMeshAttachedTransform(id, relative_location, relative_rotation)
Type | Parameter | Default | Description |
---|---|---|---|
string | id | Required parameter | Unique ID of the StaticMesh set with AddStaticMeshAttached |
Vector | relative_location | Required parameter | New relative location |
Rotator | relative_rotation | Required parameter | New relative rotation |
See also AddStaticMeshAttached.
🚀 Events
Name | Description | |
---|---|---|
CharacterAttemptEnter | Triggered when a Character attempts to enter the Vehicle | |
CharacterAttemptLeave | Triggered when a Character attempts to leave the Vehicle | |
![]() | CharacterEnter | Triggered when a Character fully enters the Vehicle |
![]() | CharacterLeave | Triggered when a Character fully leaves the Vehicle |
![]() | Hit | Triggered when Vehicle hits something |
TakeDamage | Triggered when this Vehicle takes damage |

CharacterAttemptEnter
Triggered when a Character attempts to enter the Vehicle
Return false to prevent it
Vehicle.Subscribe("CharacterAttemptEnter", function(self, character, seat)
-- CharacterAttemptEnter was called
end)
Type | Argument | Description |
---|---|---|
Base Vehicle | self | No description provided |
Character | character | No description provided |
integer | seat | The seat index |

CharacterAttemptLeave
Triggered when a Character attempts to leave the Vehicle
Return false to prevent it
Vehicle.Subscribe("CharacterAttemptLeave", function(self, character)
-- CharacterAttemptLeave was called
end)
Type | Argument | Description |
---|---|---|
Base Vehicle | self | No description provided |
Character | character | No description provided |
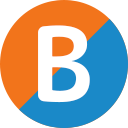
CharacterEnter
Triggered when a Character fully enters the Vehicle
Vehicle.Subscribe("CharacterEnter", function(self, character, seat)
-- CharacterEnter was called
end)
Type | Argument | Description |
---|---|---|
Base Vehicle | self | No description provided |
Character | character | No description provided |
integer | seat | The seat index |
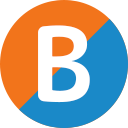
CharacterLeave
Triggered when a Character fully leaves the Vehicle
Vehicle.Subscribe("CharacterLeave", function(self, character)
-- CharacterLeave was called
end)
Type | Argument | Description |
---|---|---|
Base Vehicle | self | No description provided |
Character | character | No description provided |
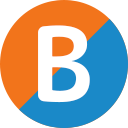
Hit
Triggered when Vehicle hits something
Vehicle.Subscribe("Hit", function(self, impact_force, normal_impulse, impact_location, velocity)
-- Hit was called
end)
Type | Argument | Description |
---|---|---|
Base Vehicle | self | No description provided |
integer | impact_force | The intensity of the Hit normalized by the Vehicle's weight |
Vector | normal_impulse | The impulse direction it hits |
Vector | impact_location | The world 3D location of the impact |
Vector | velocity | The Vehicle velocity at the moment it hits |

TakeDamage
Triggered when this Vehicle takes damage
Returnfalse
to cancel the damage (will still display animations, particles and apply impact forces)
Vehicle.Subscribe("TakeDamage", function(self, damage, bone, type, from_direction, instigator, causer)
-- TakeDamage was called
end)
Type | Argument | Description |
---|---|---|
Base Vehicle | self | No description provided |
integer | damage | No description provided |
string | bone | Damaged bone |
DamageType | type | Damage Type |
Vector | from_direction | Direction of the damage relative to the damaged actor |
Player | instigator | The player which caused the damage |
any | causer | The object which caused the damage |