Base Pickable
Pickables are special Actors which can be grabbed, held and used by Characters.
They have special methods and events and are highlighted when looked at by a Character.
🦠 Functions
Returns | Name | Description | |
---|---|---|---|
![]() | AddSkeletalMeshAttached | Attaches a Skeletal Mesh as master pose to this entity | |
![]() | AddStaticMeshAttached | Attaches a Static Mesh to this entity | |
![]() | table of string | GetAllSkeletalMeshAttached | Gets all Skeletal Meshes attached to this entity |
![]() | table of string | GetAllStaticMeshAttached | Gets all Static Meshes attached to this entity |
![]() | Character or nil | GetHandler | Gets the Character, if it exists, that's holding this Pickable |
![]() | HandlingMode | GetHandlingMode | Gets the Handling Mode of this Pickable |
![]() | SkeletalMesh Reference | GetMesh | Gets the name of the asset this Pickable uses |
PullUse | Pulls the usage of this Pickable (will start firing if this is a weapon) | ||
ReleaseUse | Releases the usage of this Pickable (will stop firing if this is a weapon) | ||
![]() | RemoveAllSkeletalMeshesAttached | Removes all SkeletalMeshes attached | |
![]() | RemoveAllStaticMeshesAttached | Removes all StaticMeshes attached | |
![]() | RemoveSkeletalMeshAttached | Removes, if it exists, a SkeletalMesh from this Pickable given its custom ID | |
![]() | RemoveStaticMeshAttached | Removes, if it exists, a StaticMesh from this Pickable given its custom ID | |
SetAttachmentSettings | Sets the Attachment Settings for this Pickable (how it attaches to the Character when Picking up) | ||
SetCrosshairMaterial | Sets the crosshair material for this Pickable | ||
SetPickable | Sets if this Pickable can be picked up from ground by the player | ||
![]() | SetStaticMeshAttachedTransform | Sets a Static Mesh Attached location and rotation |
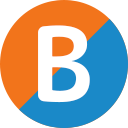
AddSkeletalMeshAttached
Spawns and attaches a SkeletalMesh to this Pickable, the SkeletalMesh must have the same skeleton used by this Actor's mesh, and will follow all animations from it. Uses a custom ID to be used for removing/customizing it afterwards
my_pickable:AddSkeletalMeshAttached(id, skeletal_mesh_path, use_parent_bounds?)
Type | Parameter | Default | Description |
---|---|---|---|
string | id | Required parameter | Used further for removing or applying material settings on it |
SkeletalMesh Reference | skeletal_mesh_path | Required parameter | Path to SkeletalMesh asset to attach |
boolean | use_parent_bounds? | true | If true, this component uses its parents bounds when attached. This can be a significant optimization with many components attached together |
See also RemoveSkeletalMeshAttached.
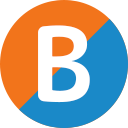
AddStaticMeshAttached
Spawns and attaches a StaticMesh to this Pickable in a Socket with a relative location and rotation. Uses a custom ID to be used for removing/customizing it afterwards
my_pickable:AddStaticMeshAttached(id, static_mesh_path, socket?, relative_location?, relative_rotation?, use_parent_bounds?)
Type | Parameter | Default | Description |
---|---|---|---|
string | id | Required parameter | Unique ID to assign to the StaticMesh |
StaticMesh Reference | static_mesh_path | Required parameter | Path to StaticMesh asset to attach |
string | socket? |
| Bone socket to attach to |
Vector | relative_location? | Vector(0, 0, 0) | Relative location |
Rotator | relative_rotation? | Rotator(0, 0, 0) | Relative rotation |
boolean | use_parent_bounds? | true | If true, this component uses its parents bounds when attached. This can be a significant optimization with many components attached together |
See also SetStaticMeshAttachedTransform, RemoveStaticMeshAttached.
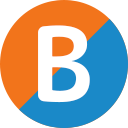
GetAllSkeletalMeshAttached
Gets all Skeletal Meshes attached to this entity
— Returns table of string (the key as the Attached ID, and the value as the Asset Path).
local ret = my_pickable:GetAllSkeletalMeshAttached()
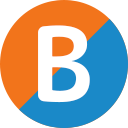
GetAllStaticMeshAttached
Gets all Static Meshes attached to this entity
— Returns table of string (the key as the Attached ID, and the value as the Asset Path).
local ret = my_pickable:GetAllStaticMeshAttached()
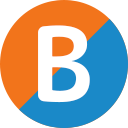
GetHandler
Gets the Character, if it exists, that's holding this Pickable
local ret = my_pickable:GetHandler()
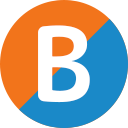
GetHandlingMode
Gets the Handling Mode of this Pickable
— Returns HandlingMode.
local ret = my_pickable:GetHandlingMode()
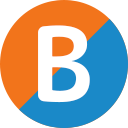
GetMesh
Gets the name of the asset this Pickable uses
— Returns SkeletalMesh Reference.
local ret = my_pickable:GetMesh()

PullUse
Pulls the usage of this Pickable (will start firing if this is a weapon)
my_pickable:PullUse(release_use_after?)
Type | Parameter | Default | Description |
---|---|---|---|
float | release_use_after? | -1 | Time in seconds to automatically release the usage (-1 will not release, 0 will release one tick after) |

ReleaseUse
Releases the usage of this Pickable (will stop firing if this is a weapon)
my_pickable:ReleaseUse()
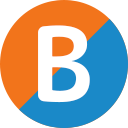
RemoveAllSkeletalMeshesAttached
Removes all SkeletalMeshes attached
my_pickable:RemoveAllSkeletalMeshesAttached()
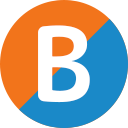
RemoveAllStaticMeshesAttached
Removes all StaticMeshes attached
my_pickable:RemoveAllStaticMeshesAttached()
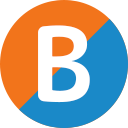
RemoveSkeletalMeshAttached
Removes, if it exists, a SkeletalMesh from this Pickable given its custom ID
my_pickable:RemoveSkeletalMeshAttached(id)
Type | Parameter | Default | Description |
---|---|---|---|
string | id | Required parameter | Unique ID of the SkeletalMesh to remove |
See also AddSkeletalMeshAttached.
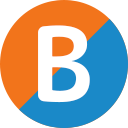
RemoveStaticMeshAttached
Removes, if it exists, a StaticMesh from this Pickable given its custom ID
my_pickable:RemoveStaticMeshAttached(id)
Type | Parameter | Default | Description |
---|---|---|---|
string | id | Required parameter | Unique ID of the StaticMesh to remove |
See also AddStaticMeshAttached.

SetAttachmentSettings
Sets the Attachment Settings for this Pickable (how it attaches to the Character when Picking up)
my_pickable:SetAttachmentSettings(relative_location, relative_rotation?, socket?)
Type | Parameter | Default | Description |
---|---|---|---|
Vector | relative_location | Required parameter | Location relative to the Socket |
Rotator | relative_rotation? | Rotator(0, 0, 0) | Rotation relative to the Socket |
string | socket? | hand_r_socket | Character Socket to attach to when picked up |

SetCrosshairMaterial
Sets the crosshair material for this Pickable
my_pickable:SetCrosshairMaterial(material_asset)
Type | Parameter | Default | Description |
---|---|---|---|
Material Reference | material_asset | Required parameter | Asset path to the crosshair material |

SetPickable
Sets if this Pickable can be picked up from ground by the player
my_pickable:SetPickable(is_pickable)
Type | Parameter | Default | Description |
---|---|---|---|
boolean | is_pickable | Required parameter | No description provided |
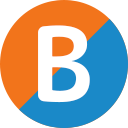
SetStaticMeshAttachedTransform
Sets a Static Mesh Attached location and rotation
my_pickable:SetStaticMeshAttachedTransform(id, relative_location, relative_rotation)
Type | Parameter | Default | Description |
---|---|---|---|
string | id | Required parameter | Unique ID of the StaticMesh set with AddStaticMeshAttached |
Vector | relative_location | Required parameter | New relative location |
Rotator | relative_rotation | Required parameter | New relative rotation |
See also AddStaticMeshAttached.
🚀 Events
Name | Description | |
---|---|---|
![]() | Drop | When a Character drops this Pickable |
![]() | Hit | When this Pickable hits something |
Interact | Triggered when a Character interacts with this Pickable (i.e. tries to pick it up) | |
![]() | PickUp | Triggered When a Character picks this up |
![]() | PullUse | Triggered when a Character presses the use button for this Pickable (i.e. clicks left mouse button with this equipped) |
![]() | ReleaseUse | Triggered when a Character releases the use button for this Pickable (i.e. releases left mouse button with this equipped) |
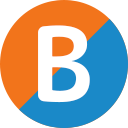
Drop
When a Character drops this Pickable
Pickable.Subscribe("Drop", function(self, character, was_triggered_by_player)
-- Drop was called
end)
Type | Argument | Description |
---|---|---|
Base Pickable | self | The Pickable which has been dropped |
Character | character | The Character that dropped it |
boolean | was_triggered_by_player | If the Player actively pressed the Drop binding to drop |
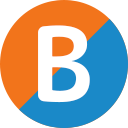
Hit
When this Pickable hits something
Pickable.Subscribe("Hit", function(self, impact_force, normal_impulse, impact_location, velocity)
-- Hit was called
end)
Type | Argument | Description |
---|---|---|
Base Pickable | self | The Actor that was hit |
float | impact_force | The intensity of the hit normalized by the Pickable's weight |
Vector | normal_impulse | The impulse direction of the hit |
Vector | impact_location | The world space location of the impact |
Vector | velocity | The Pickable's velocity at the moment it hit |

Interact
Triggered when a Character interacts with this Pickable (i.e. tries to pick it up)
Returnfalse
to prevent the interaction
Pickable.Subscribe("Interact", function(self, character)
-- Interact was called
end)
Type | Argument | Description |
---|---|---|
Base Pickable | self | The Pickable that just got interacted with |
Character | character | The Character that interacted with it |
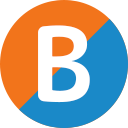
PickUp
Triggered When a Character picks this up
Pickable.Subscribe("PickUp", function(self, character)
-- PickUp was called
end)
Type | Argument | Description |
---|---|---|
Base Pickable | self | The Pickable that just got picked up |
Character | character | The Character that picked it up |
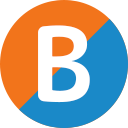
PullUse
Triggered when a Character presses the use button for this Pickable (i.e. clicks left mouse button with this equipped)
Pickable.Subscribe("PullUse", function(self, character)
-- PullUse was called
end)
Type | Argument | Description |
---|---|---|
Base Pickable | self | The Pickable which has just been used |
Character | character | The Character that used it |
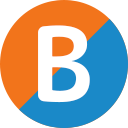
ReleaseUse
Triggered when a Character releases the use button for this Pickable (i.e. releases left mouse button with this equipped)
Pickable.Subscribe("ReleaseUse", function(self, character)
-- ReleaseUse was called
end)
Type | Argument | Description |
---|---|---|
Base Pickable | self | The Pickable which has just stopped being used |
Character | character | The Character that stopped using it |
➕ Available Crosshairs
nanos world provides a bunch of crosshair materials which can be used in Weapons/Pickables. You can of course create your own crosshair materialr and use those instead!
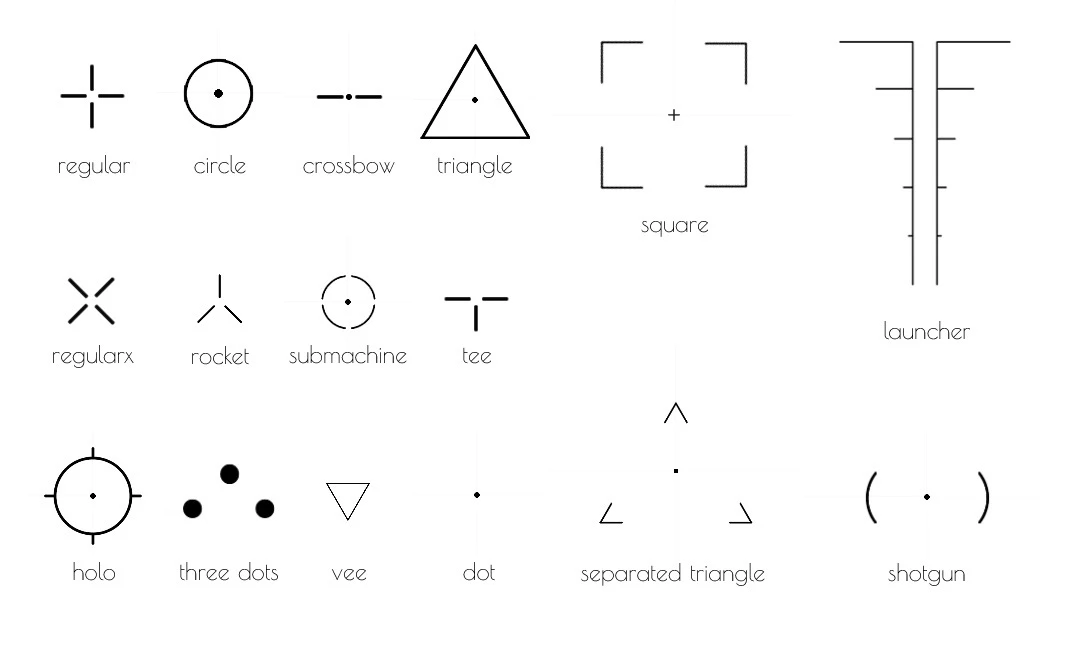
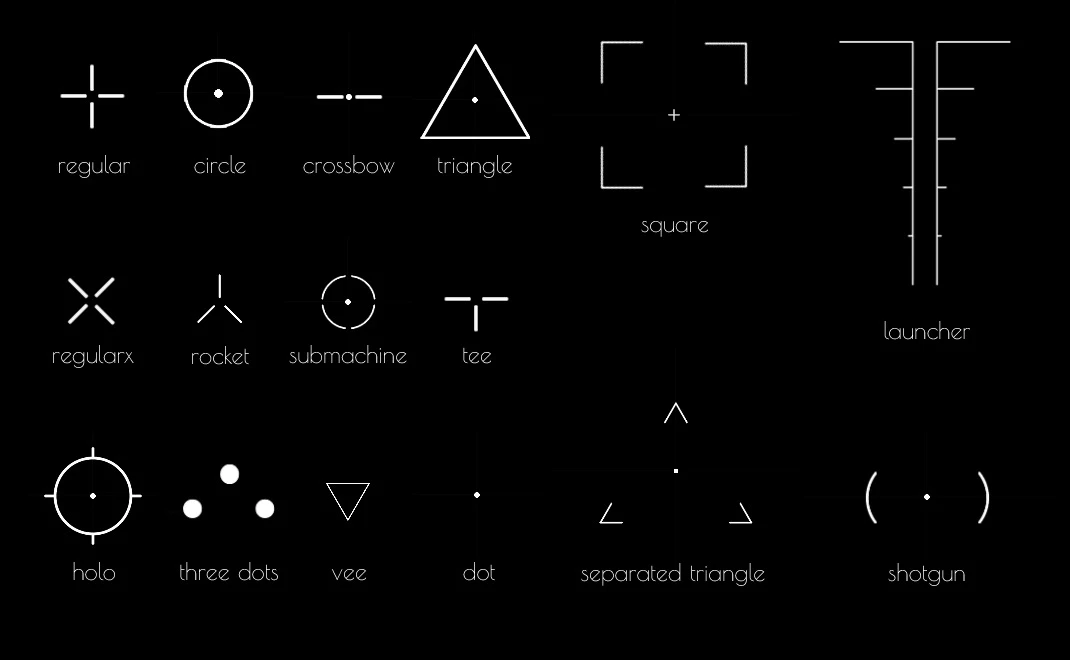
List of crosshair materials included in the default asset pack
nanos-world::MI_Crosshair_Circle
nanos-world::MI_Crosshair_Crossbow
nanos-world::MI_Crosshair_Dot
nanos-world::MI_Crosshair_Holo
nanos-world::MI_Crosshair_Launcher
nanos-world::MI_Crosshair_Regular
nanos-world::MI_Crosshair_Regular_X
nanos-world::MI_Crosshair_Rocket
nanos-world::MI_Crosshair_Separated_Triangle
nanos-world::MI_Crosshair_Shotgun
nanos-world::MI_Crosshair_Square
nanos-world::MI_Crosshair_Submachine
nanos-world::MI_Crosshair_Tee
nanos-world::MI_Crosshair_ThreeDots
nanos-world::MI_Crosshair_Triangle
nanos-world::MI_Crosshair_Vee