💬 Chat
Configure, send and intercept chat messages.
🗿Static Class
This is a Static Class. Access it's methods directly with
.
. It's not possible to spawn new instances.🧑💻API Source
This page is auto-generated! The Functions, Properties and Events described here are defined in our GitHub's API Repository! Feel free to commit suggestions and changes to the source .json API files!
🎒 Examples
(Chat.BroadcastMessage) Sends a message to everyone
Chat.BroadcastMessage("Welcome to the server!")
Colors in Chat
It is also possible to send colored messages in the Chat! For that, just circumvent a piece of text with a style tag: <TAG>my awesome text</>
.
Server/Index.lua
Chat.BroadcastMessage("Hello with <cyan>Cyan</> text message!")
All supported text styles/tags:
<cyan>
<green>
<blue>
<purple>
<marengo>
<yellow>
<orange>
<red>
<grey>
<bold>
<italic>
note
It is NOT possible to combine two or more styles together (e.g. <bold>
+ <red>
).
🗿 Static Functions
Returns | Name | Description | |
---|---|---|---|
AddMessage | Adds a chat message which will display local only | ||
BroadcastMessage | Sends a chat message to all Players | ||
Clear | Clears all messages | ||
SendMessage | Sends a chat message to a Player only | ||
SetConfiguration | Configures the Chat visuals and position | ||
SetVisibility | Sets if the Chat is visible or not |

AddMessage
Adds a chat message which will display local only
Chat.AddMessage(message)
Type | Parameter | Default | Description |
---|---|---|---|
string | message | Required parameter | No description provided |

BroadcastMessage
Sends a chat message to all Players
Chat.BroadcastMessage(message)
Type | Parameter | Default | Description |
---|---|---|---|
string | message | Required parameter | The message to send to all Players |
Chat.BroadcastMessage Examples
Sends a message to everyone
Chat.BroadcastMessage("Welcome to the server!")

Clear
Clears all messages
Chat.Clear()

SendMessage
Sends a chat message to a Player only
Chat.SendMessage(player, message)
Type | Parameter | Default | Description |
---|---|---|---|
Player | player | Required parameter | The player to receive the message |
string | message | Required parameter | The message |

SetConfiguration
Configures the Chat visuals and position
Chat.SetConfiguration(screen_location?, size?, anchors_min?, anchors_max?, alignment?, justify?, show_scrollbar?)
Type | Parameter | Default | Description |
---|---|---|---|
Vector2D | screen_location? | Vector2D(-25, 0) | No description provided |
Vector2D | size? | Vector2D(600, 250) | No description provided |
Vector2D | anchors_min? | Vector2D(1, 0.5) | No description provided |
Vector2D | anchors_max? | Vector2D(1, 0.5) | No description provided |
Vector2D | alignment? | Vector2D(1, 0.5) | No description provided |
boolean | justify? | true | No description provided |
boolean | show_scrollbar? | true | No description provided |

SetVisibility
Sets if the Chat is visible or not
Chat.SetVisibility(is_visible)
Type | Parameter | Default | Description |
---|---|---|---|
boolean | is_visible | Required parameter | No description provided |
🚀 Events
Name | Description | |
---|---|---|
ChatEntry | Called when a new Chat Message is received, this is also triggered when new messages are sent programatically | |
Close | When player closes the Chat | |
Open | When player opens the Chat | |
![]() | PlayerSubmit | Called when a player submits a message in the chat |

ChatEntry
Called when a new Chat Message is received, this is also triggered when new messages are sent programatically
This is useful for creating your own Chat interface while still use the built-in system
Chat.Subscribe("ChatEntry", function(message, player)
-- ChatEntry was called
end)
Type | Argument | Description |
---|---|---|
string | message | The message |
Player or nil | player | The player who sent the message or nil if this was called on client side or was sent through code |

Close
When player closes the Chat
Chat.Subscribe("Close", function()
-- Close was called
end)

Open
When player opens the Chat
Chat.Subscribe("Open", function()
-- Open was called
end)
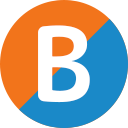
PlayerSubmit
Called when a player submits a message in the chat
Return false to prevent the message from being sent
Chat.Subscribe("PlayerSubmit", function(message, player)
-- PlayerSubmit was called
end)