👩💻 Player
Players are Entities that represents the individual behind the mouse and keyboard. Players are spawned automatically when connected to the server.
🟥 You cannot spawn or Destroy Players.
🎒 Examples
-- Spawns and possess a Character when a Player joins the server
Player.Subscribe("Spawn", function(player)
local new_char = Character(Vector(), Rotator(), "nanos-world::SK_Male")
player:Possess(new_char)
end)
-- Destroys the Character when the Player leaves the server
Player.Subscribe("Destroy", function(player)
local character = player:GetControlledCharacter()
if (character) then
character:Destroy()
end
end)
🗿 Static Functions
Inherited Entity Static Functions
Base Entityscripting-reference/classes/base-classes/Entity
Returns | Name | Description | |
---|---|---|---|
![]() | table of Base Entity | GetAll | Returns a table containing all Entities of the class this is called on |
![]() | Base Entity | GetByIndex | Returns a specific Entity of this class at an index |
![]() | integer | GetCount | Returns how many Entities of this class exist |
![]() | table of table | GetInheritedClasses | Gets a list of all directly inherited classes from this Class created with the Inheriting System |
![]() | iterator | GetPairs | Returns an iterator with all Entities of this class to be used with pairs() |
![]() | table or nil | GetParentClass | Gets the parent class if this Class was created with the Inheriting System |
![]() | table | Inherit | Inherits this class with the Inheriting System |
![]() | boolean | IsChildOf | Gets if this Class is child of another class if this Class was created with the Inheriting System |
![]() | function | Subscribe | Subscribes to an Event for all entities of this Class |
![]() | function | SubscribeRemote | Subscribes to a custom event called from server |
![]() | Unsubscribe | Unsubscribes all callbacks from this Event in this Class within this Package, or only the callback passed |
This class doesn't have own static functions.
🦠 Functions
Inherited Entity Functions
Base Entityscripting-reference/classes/base-classes/Entity
Returns | Name | Description | |
---|---|---|---|
BroadcastRemoteEvent | Calls a custom remote event directly on this entity to all Players | ||
CallRemoteEvent | Calls a custom remote event directly on this entity to a specific Player | ||
CallRemoteEvent | Calls a custom remote event directly on this entity | ||
Destroy | Destroys this Entity | ||
table of string | GetAllValuesKeys | Gets a list of all values keys | |
![]() | table | GetClass | Gets the class of this entity |
![]() | integer | GetID | Gets the universal network ID of this Entity (same on both client and server) |
![]() | any | GetValue | Gets a Value stored on this Entity at the given key |
![]() | boolean | IsA | Recursively checks if this entity is inherited from a Class |
![]() | boolean | IsValid | Returns true if this Entity is valid (i.e. wasn't destroyed and points to a valid Entity) |
![]() | SetValue | Sets a Value in this Entity | |
![]() | function | Subscribe | Subscribes to an Event on this specific entity |
![]() | function | SubscribeRemote | Subscribes to a custom event called from server on this specific entity |
![]() | Unsubscribe | Unsubscribes all callbacks from this Event in this Entity within this Package, or only the callback passed |
Returns | Name | Description | |
---|---|---|---|
![]() | AttachCameraTo | Attaches the Player`s Camera to an Actor | |
Ban | Bans the player from the server | ||
Connect | Redirects the player to another server | ||
![]() | string | GetAccountIconURL | Return a URL which can be used through WebUI and Widgets to display the Player's Steam Avatar (64x64) |
![]() | string | GetAccountID | No description provided |
![]() | string | GetAccountName | No description provided |
float | GetCameraArmLength | No description provided | |
Vector | GetCameraLocation | No description provided | |
Rotator | GetCameraRotation | No description provided | |
![]() | Character or nil | GetControlledCharacter | No description provided |
![]() | integer | GetDimension | Gets this Player's dimension |
string | GetIP | No description provided | |
![]() | string | GetName | No description provided |
![]() | integer | GetPing | No description provided |
![]() | string | GetSteamID | No description provided |
![]() | integer | GetVOIPChannel | No description provided |
![]() | VOIPSetting | GetVOIPSetting | No description provided |
boolean | IsHost | No description provided | |
boolean | IsLocalPlayer | No description provided | |
Kick | Kicks the player from the server | ||
Possess | Makes a Player to possess and control a Pawn | ||
![]() | ResetCamera | Resets the Camera to default state (Unspectate and Detaches) | |
![]() | RotateCameraTo | Smoothly moves the Player's Camera Rotation | |
![]() | SetCameraArmLength | Sets the Player's Camera Arm Length (Spring Arm length) | |
![]() | SetCameraFOV | Sets the Player's Camera FOV (if not possessing a Character) | |
![]() | SetCameraLocation | Sets the Player's Camera Location (only works if not possessing any Character) | |
![]() | SetCameraRotation | Sets the Player's Camera Rotation | |
![]() | SetCameraSocketOffset | Sets the Player's Camera Socket Offset (Spring Arm Offset) | |
![]() | SetCameraSpeedSettings | Sets the Player's Camera Speed Settings (if not possessing a Character) | |
SetDimension | Sets this Player's dimension | ||
![]() | SetManualCameraFade | Turns on camera fading at the given opacity | |
SetName | Sets the player's name | ||
SetVOIPChannel | Sets the VOIP Channel of this Player (will only communicate with other players in the same channel) | ||
![]() | SetVOIPSetting | Sets the VOIP Settings of this Player | |
![]() | SetVOIPVolume | Sets the VOIP Volume of this Player | |
![]() | Spectate | Spectates other Player | |
![]() | StartCameraFade | Does a camera fade to/from a solid color | |
![]() | StopCameraFade | Stops camera fading. | |
![]() | TranslateCameraTo | Smoothly moves the Player's Camera Location (only works if not possessing any Character) | |
UnPossess | Release the Player from the Character |
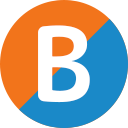
AttachCameraTo
Attaches the Player`s Camera to an Actor
my_player:AttachCameraTo(actor, socket_offset, blend_speed)
Type | Parameter | Default | Description |
---|---|---|---|
Base Actor | actor | Required parameter | No description provided |
Vector | socket_offset | Required parameter | No description provided |
float | blend_speed | Required parameter | No description provided |

Ban
Bans the player from the server
my_player:Ban(reason)
Type | Parameter | Default | Description |
---|---|---|---|
string | reason | Required parameter | No description provided |

Connect
Redirects the player to another server
my_player:Connect(IP, password?)
Type | Parameter | Default | Description |
---|---|---|---|
string | IP | Required parameter | No description provided |
string | password? |
| Server password |
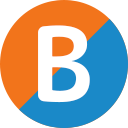
GetAccountIconURL
Return a URL which can be used through WebUI and Widgets to display the Player's Steam Avatar (64x64)
— Returns string.
local ret = my_player:GetAccountIconURL()
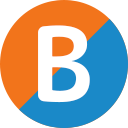
GetAccountID
— Returns string.
local ret = my_player:GetAccountID()
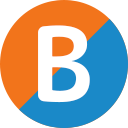
GetAccountName
— Returns string.
local ret = my_player:GetAccountName()

GetCameraArmLength
— Returns float.
local ret = my_player:GetCameraArmLength(return_base?)
Type | Parameter | Default | Description |
---|---|---|---|
boolean | return_base? | false | Whether to return the current (false) or base (true) value. The base is the same value set by SetCameraArmLength(). |
See also SetCameraArmLength.

GetCameraLocation
— Returns Vector.
local ret = my_player:GetCameraLocation()
See also SetCameraLocation.

GetCameraRotation
— Returns Rotator.
local ret = my_player:GetCameraRotation()
See also SetCameraRotation.
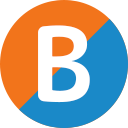
GetControlledCharacter
local ret = my_player:GetControlledCharacter()
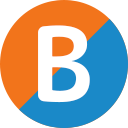
GetDimension
Gets this Player's dimension
— Returns integer.
local ret = my_player:GetDimension()
See also SetDimension, DimensionChange.

GetIP
— Returns string.
local ret = my_player:GetIP()
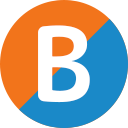
GetName
— Returns string.
local ret = my_player:GetName()
See also SetName.
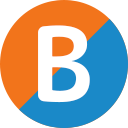
GetPing
— Returns integer.
local ret = my_player:GetPing()
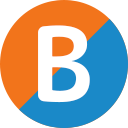
GetSteamID
— Returns string.
local ret = my_player:GetSteamID()
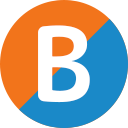
GetVOIPChannel
— Returns integer.
local ret = my_player:GetVOIPChannel()
See also SetVOIPChannel.
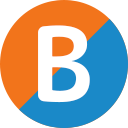
GetVOIPSetting
— Returns VOIPSetting.
local ret = my_player:GetVOIPSetting()
See also SetVOIPSetting.

IsHost
— Returns boolean.
local ret = my_player:IsHost()

IsLocalPlayer
— Returns boolean.
local ret = my_player:IsLocalPlayer()

Kick
Kicks the player from the server
my_player:Kick(reason)
Type | Parameter | Default | Description |
---|---|---|---|
string | reason | Required parameter | No description provided |

Possess
Makes a Player to possess and control a Pawn
my_player:Possess(new_pawn, blend_time?, exp?)
Type | Parameter | Default | Description |
---|---|---|---|
Base Pawn | new_pawn | Required parameter | No description provided |
float | blend_time? | 0 | No description provided |
float | exp? | 0 | No description provided |
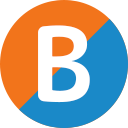
ResetCamera
Resets the Camera to default state (Unspectate and Detaches)
my_player:ResetCamera()
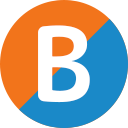
RotateCameraTo
Smoothly moves the Player's Camera Rotation
my_player:RotateCameraTo(rotation, time, exp?)
Type | Parameter | Default | Description |
---|---|---|---|
Rotator | rotation | Required parameter | No description provided |
float | time | Required parameter | No description provided |
float | exp? | 0 | Exponential used to smooth interp, use 0 for linear movement |
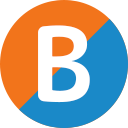
SetCameraArmLength
Sets the Player's Camera Arm Length (Spring Arm length)
my_player:SetCameraArmLength(length, force)
Type | Parameter | Default | Description |
---|---|---|---|
float | length | Required parameter | No description provided |
boolean | force | Required parameter | Whether to bypass interpolation and set the target to its value directly |
See also GetCameraArmLength.
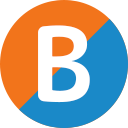
SetCameraFOV
Sets the Player's Camera FOV (if not possessing a Character)
my_player:SetCameraFOV(fov?)
Type | Parameter | Default | Description |
---|---|---|---|
float | fov? | 90 | Value must be between 5 and 170. Pass empty to reset to default. |
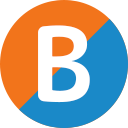
SetCameraLocation
Sets the Player's Camera Location (only works if not possessing any Character)
my_player:SetCameraLocation(location)
Type | Parameter | Default | Description |
---|---|---|---|
Vector | location | Required parameter | No description provided |
See also GetCameraLocation.
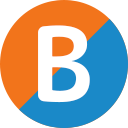
SetCameraRotation
Sets the Player's Camera Rotation
my_player:SetCameraRotation(rotation)
Type | Parameter | Default | Description |
---|---|---|---|
Rotator | rotation | Required parameter | No description provided |
See also GetCameraRotation.
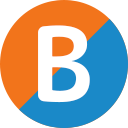
SetCameraSocketOffset
Sets the Player's Camera Socket Offset (Spring Arm Offset)
my_player:SetCameraSocketOffset(socket_offset)
Type | Parameter | Default | Description |
---|---|---|---|
Vector | socket_offset | Required parameter | No description provided |
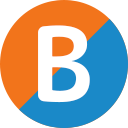
SetCameraSpeedSettings
Sets the Player's Camera Speed Settings (if not possessing a Character)
my_player:SetCameraSpeedSettings(max_speed?, acceleration?, deceleration?, turning_boost?)
Type | Parameter | Default | Description |
---|---|---|---|
float | max_speed? | 1200 | Maximum velocity magnitude allowed |
float | acceleration? | 4000 | Acceleration applied by input (rate of change of velocity) |
float | deceleration? | 4000 | Deceleration applied when there is no input (rate of change of velocity) |
float | turning_boost? | 8 | Setting affecting extra force applied when changing direction, making turns have less drift and become more responsive. Velocity magnitude is not allowed to increase, that only happens due to normal acceleration. It may decrease with large direction changes. Larger values apply extra force to reach the target direction more quickly, while a zero value disables any extra turn force. |

SetDimension
Sets this Player's dimension
my_player:SetDimension(dimension)
Type | Parameter | Default | Description |
---|---|---|---|
integer | dimension | Required parameter | No description provided |
See also GetDimension, DimensionChange.
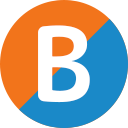
SetManualCameraFade
Turns on camera fading at the given opacity. Does not auto-animate, allowing user to animate themselves. Call StopCameraFade to turn fading back off.
my_player:SetManualCameraFade(in_fade_amount, color, in_fade_audio)
Type | Parameter | Default | Description |
---|---|---|---|
float | in_fade_amount | Required parameter | Range [0..1], where 0 is fully transparent and 1 is fully opaque solid color. |
Color | color | Required parameter | No description provided |
boolean | in_fade_audio | Required parameter | No description provided |

SetName
Sets the player's name
my_player:SetName(player_name)
Type | Parameter | Default | Description |
---|---|---|---|
string | player_name | Required parameter | No description provided |
See also GetName.

SetVOIPChannel
Sets the VOIP Channel of this Player (will only communicate with other players in the same channel)
my_player:SetVOIPChannel(channel)
Type | Parameter | Default | Description |
---|---|---|---|
integer | channel | Required parameter | No description provided |
See also GetVOIPChannel.
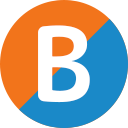
SetVOIPSetting
Sets the VOIP Settings of this Player
my_player:SetVOIPSetting(voip_setting)
Type | Parameter | Default | Description |
---|---|---|---|
VOIPSetting | voip_setting | Required parameter | No description provided |
See also GetVOIPSetting.
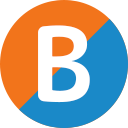
SetVOIPVolume
Sets the VOIP Volume of this Player
my_player:SetVOIPVolume(volume)
Type | Parameter | Default | Description |
---|---|---|---|
float | volume | Required parameter | No description provided |
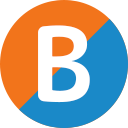
Spectate
Spectates other Player
my_player:Spectate(player, blend_speed?)
Type | Parameter | Default | Description |
---|---|---|---|
Player | player | Required parameter | No description provided |
float | blend_speed? | 0 | No description provided |
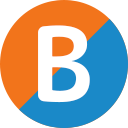
StartCameraFade
Does a camera fade to/from a solid color. Animates automatically
my_player:StartCameraFade(from_alpha, to_alpha, duration, to_color, should_fade_audio?, hold_when_finished?)
Type | Parameter | Default | Description |
---|---|---|---|
float | from_alpha | Required parameter | Alpha at which to begin the fade. Range [0..1], where 0 is fully transparent and 1 is fully opaque solid color. |
float | to_alpha | Required parameter | Alpha at which to finish the fade. |
float | duration | Required parameter | How long the fade should take, in seconds. |
Color | to_color | Required parameter | Color to fade to/from. |
boolean | should_fade_audio? |
| True to fade audio volume along with the alpha of the solid color. |
boolean | hold_when_finished? |
| True for fade to hold at the ToAlpha until explicitly stopped (e.g. with StopCameraFade) |
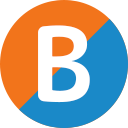
StopCameraFade
Stops camera fading.
my_player:StopCameraFade()
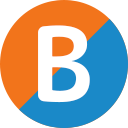
TranslateCameraTo
Smoothly moves the Player's Camera Location (only works if not possessing any Character)
my_player:TranslateCameraTo(location, time, exp?)
Type | Parameter | Default | Description |
---|---|---|---|
Vector | location | Required parameter | No description provided |
float | time | Required parameter | No description provided |
float | exp? | 0 | Exponential used to smooth interp, use 0 for linear movement |

UnPossess
Release the Player from the Character
my_player:UnPossess()
🚀 Events
Inherited Entity Events
Base Entityscripting-reference/classes/base-classes/Entity
Name | Description | |
---|---|---|
![]() | ClassRegister | Triggered when a new Class is registered with the Inheriting System |
![]() | Destroy | Triggered when an Entity is destroyed |
![]() | Spawn | Triggered when an Entity is spawned/created |
![]() | ValueChange | Triggered when an Entity has a value changed with :SetValue() |
Name | Description | |
---|---|---|
DimensionChange | Triggered when a Player changes it's dimension | |
![]() | Possess | Trigerred when Player starts controlling a Character |
Ready | Triggered when Player is ready (the client fully joined, loaded the map and all entities and is ready to play) | |
![]() | UnPossess | A Character was released from the Player |
![]() | VOIP | When a Player starts/ends using VOIP |

DimensionChange
Triggered when a Player changes it's dimension
Player.Subscribe("DimensionChange", function(self, old_dimension, new_dimension)
-- DimensionChange was called
end)
Type | Argument | Description |
---|---|---|
Player | self | No description provided |
integer | old_dimension | No description provided |
integer | new_dimension | No description provided |
See also SetDimension, GetDimension.
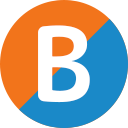
Possess
Trigerred when Player starts controlling a Character
Player.Subscribe("Possess", function(self, character)
-- Possess was called
end)
See also GetControlledCharacter.

Ready
Triggered when Player is ready (the client fully joined, loaded the map and all entities and is ready to play)
Player.Subscribe("Ready", function(self)
-- Ready was called
end)
Type | Argument | Description |
---|---|---|
Player | self | No description provided |
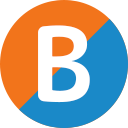
UnPossess
A Character was released from the Player
Player.Subscribe("UnPossess", function(self, character)
-- UnPossess was called
end)
See also GetControlledCharacter.
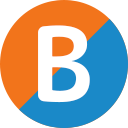
VOIP
When a Player starts/ends using VOIP
When 'is_talking' is true, return false to prevent this voice stream from playing (on server will prevent for everyone, on client will prevent only for the client)
Player.Subscribe("VOIP", function(self, is_talking)
-- VOIP was called
end)