⏱️ Timer
Execute code at specified time intervals.
🗿Static Class
This is a Static Class. Access it's methods directly with
.
. It's not possible to spawn new instances.🧑💻API Source
This page is auto-generated! The Functions, Properties and Events described here are defined in our GitHub's API Repository! Feel free to commit suggestions and changes to the source .json API files!
info
The shortest interval possible is equal to the local Tick Rate - usually at 33ms. On the Server this can vary depending on the Config.toml setting.
🎒 Examples
-- creates a Interval to call a function at every 2 seconds
local my_interval = Timer.SetInterval(function(param1, param2)
Console.Log("Triggered each 2 seconds! Param1: " .. param1 .. ". Param2: " .. param2)
end, 2000, "awesome param 1", 456)
-- cancels the Interval
Timer.ClearInterval(my_interval)
-- creates a Timeout to call my_function in 5 seconds, once - passing a parameter
Timer.SetTimeout(function(my_param)
Console.Log("nanos " .. my_param)
end, 5000, "world")
(Timer.Bind) Binding a Timer to an Entity
local character = Character(Vector(), Rotator(), "nanos-world::SK_Male")
local my_timer = Timer.SetTimeout(function(_character)
-- Do something with _character
-- Ex: Destroy the character after 10 seconds
_character:Destroy()
end, 10000, character)
-- Binds the Timer to the Character
-- This will ensure it will never trigger if the character is destroyed before it
-- With this you don't need to validate if the '_character' parameter is valid
Timer.Bind(my_timer, character)
🗿 Static Functions
Returns | Name | Description | |
---|---|---|---|
![]() | Bind | Binds a Timer to any Actor. The timer will be automatically cleared when the Actor is destroyed | |
![]() | ClearInterval | Stops the execution of the function specified in SetInterval() | |
![]() | ClearTimeout | Stops the execution of the function specified in SetTimeout() | |
![]() | integer | GetElapsedTime | Returns the time elapsed since the last tick |
![]() | integer | GetRemainingTime | Returns the time remaining to the next tick |
![]() | boolean | IsValid | Checks if a Timer is currently active or waiting to be triggered |
![]() | Pause | Pauses the Timer | |
![]() | ResetElapsedTime | Resets a Timer to restart from beginning | |
![]() | Resume | Resumes the Timer | |
![]() | integer | SetInterval | Same as SetTimeout(), but repeats the execution of the function continuously |
![]() | integer | SetTimeout | Executes a function, after waiting a specified number of milliseconds |
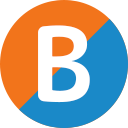
Bind
Binds a Timer to any Actor. The timer will be automatically cleared when the Actor is destroyed
Timer.Bind(timer_id, actor)
Type | Parameter | Default | Description |
---|---|---|---|
integer | timer_id | Required parameter | The Timer ID |
Base Actor | actor | Required parameter | Actor to be bound |
Timer.Bind Examples
Binding a Timer to an Entity
local character = Character(Vector(), Rotator(), "nanos-world::SK_Male")
local my_timer = Timer.SetTimeout(function(_character)
-- Do something with _character
-- Ex: Destroy the character after 10 seconds
_character:Destroy()
end, 10000, character)
-- Binds the Timer to the Character
-- This will ensure it will never trigger if the character is destroyed before it
-- With this you don't need to validate if the '_character' parameter is valid
Timer.Bind(my_timer, character)
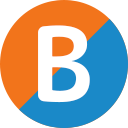
ClearInterval
Stops the execution of the function specified in SetInterval()
Timer.ClearInterval(interval_id)
Type | Parameter | Default | Description |
---|---|---|---|
integer | interval_id | Required parameter | The ID value returned by SetInterval() is used as the parameter for this method |
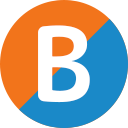
ClearTimeout
Stops the execution of the function specified in SetTimeout()
Timer.ClearTimeout(timeout_id)
Type | Parameter | Default | Description |
---|---|---|---|
integer | timeout_id | Required parameter | The ID value returned by SetTimeout() is used as the parameter for this method |
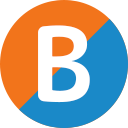
GetElapsedTime
Returns the time elapsed since the last tick
— Returns integer.
local ret = Timer.GetElapsedTime(timer_id)
Type | Parameter | Default | Description |
---|---|---|---|
integer | timer_id | Required parameter | The Timer ID |
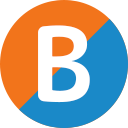
GetRemainingTime
Returns the time remaining to the next tick
— Returns integer.
local ret = Timer.GetRemainingTime(timer_id)
Type | Parameter | Default | Description |
---|---|---|---|
integer | timer_id | Required parameter | The Timer ID |
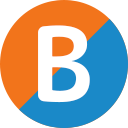
IsValid
Checks if a Timer is currently active or waiting to be triggered
— Returns boolean.
local ret = Timer.IsValid(timer_id)
Type | Parameter | Default | Description |
---|---|---|---|
integer | timer_id | Required parameter | The Timer ID |
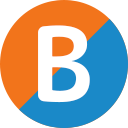
Pause
Pauses the Timer
Timer.Pause(timer_id)
Type | Parameter | Default | Description |
---|---|---|---|
integer | timer_id | Required parameter | The Timer ID |
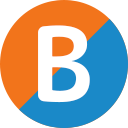
ResetElapsedTime
Resets a Timer to restart from beginning
Timer.ResetElapsedTime(timer_id)
Type | Parameter | Default | Description |
---|---|---|---|
integer | timer_id | Required parameter | The Timer ID |
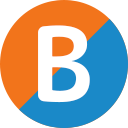
Resume
Resumes the Timer
Timer.Resume(timer_id)
Type | Parameter | Default | Description |
---|---|---|---|
integer | timer_id | Required parameter | The Timer ID |
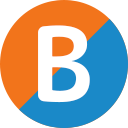
SetInterval
Same as SetTimeout(), but repeats the execution of the function continuously
— Returns integer (the interval_id).
local ret = Timer.SetInterval(callback, milliseconds?, parameters...?)
Type | Parameter | Default | Description |
---|---|---|---|
function | callback | Required parameter | The callback that will be executed. Return false to stop it from being called. |
integer | milliseconds? | 0 | The time in milliseconds the timer should delay in between executions of the specified function |
any | parameters...? | nil | Additional parameters to pass to the function |
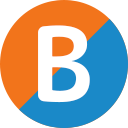
SetTimeout
Executes a function, after waiting a specified number of milliseconds
— Returns integer (the timeout_id).
local ret = Timer.SetTimeout(callback, milliseconds?, parameters...?)
Type | Parameter | Default | Description |
---|---|---|---|
function | callback | Required parameter | The callback that will be executed |
integer | milliseconds? | 0 | The time in milliseconds to wait before executing the function |
any | parameters...? | nil | Additional parameters to pass to the function |