๐ HTTP
HTTP Requests Interface.
๐ฟStatic Class
This is a Static Class. Access it's methods directly with
.
. It's not possible to spawn new instances.๐งโ๐ปAPI Source
This page is auto-generated! The Functions, Properties and Events described here are defined in our GitHub's API Repository! Feel free to commit suggestions and changes to the source .json API files!
๐ Examplesโ
Server/Index.lua
-- Makes an asynchronous HTTP Request
HTTP.RequestAsync("127.0.0.1:7777", "/", HTTPMethod.GET, "", "application/json", false, {}, function(status, data)
Console.Log(status) -- 200
Console.Log(data) -- "{"players_count":0,"server_name":"nanos world server"}"
-- TIP: You can parse it if it's a json return as well
local json_ret = JSON.parse(data)
end)
Server/Index.lua
-- Makes a synchronous HTTP Request
local ret = HTTP.Request("127.0.0.1:7777", "/", HTTPMethod.GET, "", "application/json", false, {})
Console.Log(ret.Status) -- 200
Console.Log(ret.Data) -- "{"players_count":0,"server_name":"nanos world server"}"
tip
All requests are thread safe! ๐ฅณ This means that callbacks are returned on the main thread!
๐ฟ Static Functionsโ
Returns | Name | Description | |
---|---|---|---|
table | Request | Makes a synchronous HTTP Request. The request will be made synchronously and will freeze the server until it's done. | |
![]() | RequestAsync | Makes an asynchronous HTTP Request | |
![]() | SetConnectionTimeout | Sets the global Connection Timeout in seconds | |
![]() | SetReadWriteTimeout | Sets the global Read and Write Timeout in seconds |

Request
Makes a synchronous HTTP Request.
The request will be made synchronously and will freeze the server until it's done.
โ Returns table (with this format).
local ret = HTTP.Request(uri, endpoint?, method?, data?, content_type?, compress?, headers?)
Type | Parameter | Default | Description |
---|---|---|---|
string | uri | Required parameter | The main URI (the base address) |
string | endpoint? |
| The endpoint |
HTTPMethod | method? | HTTPMethod.GET | The HTTP Method to be used |
string | data? |
| Payload |
string | content_type? | application/json | The Content Type to be used |
boolean | compress? | false | Whether or not to compress the content with gzip |
table | headers? | {} | The Headers to be used |
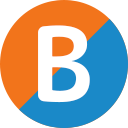
RequestAsync
Makes an asynchronous HTTP Request.
The request will be made asynchronously and returned safetly in the same thread in the callback provided when it's done.
Note: If a request is still running when unloading packages, the server will freeze until it's finished, then the package will unload.
HTTP.RequestAsync(uri, endpoint?, method?, data?, content_type?, compress?, headers?, callback?)
Type | Parameter | Default | Description |
---|---|---|---|
string | uri | Required parameter | The main URI (the base address) |
string | endpoint? |
| The endpoint |
HTTPMethod | method? | HTTPMethod.GET | The HTTP Method to be used |
string | data? |
| Payload |
string | content_type? | application/json | The Content Type to be used |
boolean | compress? | false | Whether or not to compress the content with gzip |
table | headers? | {} | The Headers to be used |
function | callback? | nil | The result with this format |
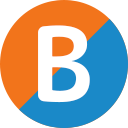
SetConnectionTimeout
Sets the global Connection Timeout in seconds
HTTP.SetConnectionTimeout(connection_timeout)
Type | Parameter | Default | Description |
---|---|---|---|
integer | connection_timeout | Required parameter | The timeout in seconds |
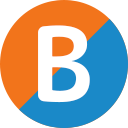
SetReadWriteTimeout
Sets the global Read and Write Timeout in seconds
HTTP.SetReadWriteTimeout(read_write_timeout)
Type | Parameter | Default | Description |
---|---|---|---|
integer | read_write_timeout | Required parameter | The timeout in seconds |
๐ Eventsโ
This class doesn't have own events.