๐ฉ Events
Subscribe for user-defined Events.
๐ฟStatic Class
This is a Static Class. Access it's methods directly with
.
. It's not possible to spawn new instances.๐งโ๐ปAPI Source
This page is auto-generated! The Functions, Properties and Events described here are defined in our GitHub's API Repository! Feel free to commit suggestions and changes to the source .json API files!
๐ Examplesโ
info
Remote means the other side, e.g.: if I'm the Client, the remote is the Server. If I'm the Server, the remote is the Client.
Basic Usageโ
Client/Index.lua
-- register for a local Event (local = client only)
Events.Subscribe("MyLocalEvent", function(my_text)
Console.Log("Event received locally! " .. my_text)
-- outputs "Event received locally! hello nanos world!"
end)
-- calls a local Event in all Local Packages
Events.Call("MyLocalEvent", "hello nanos world!")
-- register for a server Event (remote = server)
Events.SubscribeRemote("MyClientEvent", function(my_text)
Console.Log("Event received from server! " .. my_text)
-- outputs "Event received from server! hello nanos world!"
end)
-- calls a remote Event in all Server Packages
Events.CallRemote("MyServerEvent", "hello nanos world!")
info
On Server, registering for remote events has an addition parameter: Player, which is the client who sent the event.
Server/Index.lua
-- register for a local Event (local = server only)
Events.Subscribe("MyLocalEvent", function(my_text)
Console.Log("Event received locally! " .. my_text)
-- outputs "Event received locally! hello nanos world!"
end)
-- calls a local Event in all Local Packages
Events.Call("MyLocalEvent", "hello nanos world!")
-- register for a client Event (remote = client)
Events.SubscribeRemote("MyServerEvent", function(player, my_text)
Console.Log(player:GetName() .. " sent an event from client! " .. my_text)
-- outputs "Syed sent an event from client! hello nanos world!"
-- sends an "answer" to the player which sent this event
Events.CallRemote("MyClientEvent", player, "hello nanos world! message only for you!")
end)
-- sends a remote Event to all Players in all Client Packages
Events.BroadcastRemote("MyClientEvent", "hello nanos world!")
Passing entities through Eventsโ
-- register for an Event (remote or local)
Events.Subscribe("MyAnotherEvent", function(my_text, my_vector, my_character, my_number)
Console.Log("Event received! " .. my_text .. " " .. my_vector.X .. " " .. my_character:GetViewMode() .. " " .. my_number)
-- outputs "Event received! hello nanos world! 123 1 456"
end)
-- passing Characters through events
local my_temp_character = Character()
-- calls a local Event in all Local Packages
Events.Call("MyEvent", "hello nanos world!", Vector(123, 123, 123), my_temp_character, 456)
-- calls a remote Event in all Server Packages
Events.CallRemote("MyEvent", "hello nanos world!", Vector(123, 123, 123), my_temp_character, 456)
tip
You can find more examples and a compreensive guide at the Events Guide page.
๐ฟ Static Functionsโ
Returns | Name | Description | |
---|---|---|---|
BroadcastRemote | Calls an Event on Server which will be triggered in all Client's Packages of all Players | ||
BroadcastRemoteDimension | Calls an Event on Server which will be triggered in all Client's Packages of all Players in that dimension | ||
![]() | Call | Calls an Event which will be triggered in all Local Packages | |
CallRemote | Calls an Event if on Client which will be triggered in all Server Packages | ||
CallRemote | Calls an Event if on Server which will be triggered in all Client's Packages of a specific Player | ||
![]() | function | Subscribe | Subscribes for an user-created event which will be triggered for only local called events |
![]() | function | SubscribeRemote | Subscribes for an user-created event which will be triggered for only remote called events |
![]() | Unsubscribe | Unsubscribes from all subscribed events in this Package with that event name, optionally passing the function to unsubscribe only that callback | |
![]() | UnsubscribeRemote | Unsubscribes from all subscribed remote events in this Package with that event name, optionally passing the function to unsubscribe only that callback |

BroadcastRemote
Calls an Event on Server which will be triggered in all Client's Packages of all Players
Events.BroadcastRemote(event_name, args...?)
Type | Parameter | Default | Description |
---|---|---|---|
string | event_name | Required parameter | The Event Name to trigger the event |
any | args...? | nil | Arguments to pass to the event |

BroadcastRemoteDimension
Calls an Event on Server which will be triggered in all Client's Packages of all Players in that dimension
Events.BroadcastRemoteDimension(dimension, event_name, args...?)
Type | Parameter | Default | Description |
---|---|---|---|
integer | dimension | Required parameter | The Dimension to send this event |
string | event_name | Required parameter | The Event Name to trigger the event |
any | args...? | nil | Arguments to pass to the event |
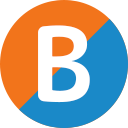
Call
Calls an Event which will be triggered in all Local Packages
Events.Call(event_name, args...?)
Type | Parameter | Default | Description |
---|---|---|---|
string | event_name | Required parameter | The Event Name to trigger the event |
any | args...? | nil | Arguments to pass to the event |

CallRemote
Calls an Event if on Client which will be triggered in all Server Packages
Events.CallRemote(event_name, args...?)
Type | Parameter | Default | Description |
---|---|---|---|
string | event_name | Required parameter | The Event Name to trigger the event |
any | args...? | nil | Arguments to pass to the event |

CallRemote
Calls an Event if on Server which will be triggered in all Client's Packages of a specific Player
Events.CallRemote(event_name, player, args...?)
Type | Parameter | Default | Description |
---|---|---|---|
string | event_name | Required parameter | The Event Name to trigger the event |
Player | player | Required parameter | The remote player to send this event |
any | args...? | nil | Arguments to pass to the event |
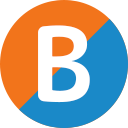
Subscribe
Subscribes for an user-created event which will be triggered for only local called events
โ Returns function (the subscribed callback itself).
local ret = Events.Subscribe(event_name, callback)
Type | Parameter | Default | Description |
---|---|---|---|
string | event_name | Required parameter | The Event Name to subscribe |
function | callback | Required parameter | The callback function to execute |
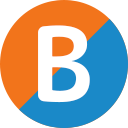
SubscribeRemote
Subscribes for an user-created event which will be triggered for only remote called events
โ Returns function (the subscribed callback itself).
local ret = Events.SubscribeRemote(event_name, callback)
Type | Parameter | Default | Description |
---|---|---|---|
string | event_name | Required parameter | The Event Name to subscribe |
function | callback | Required parameter | The callback function to execute |
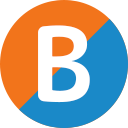
Unsubscribe
Unsubscribes from all subscribed events in this Package with that event name, optionally passing the function to unsubscribe only that callback
Events.Unsubscribe(event_name, callback?)
Type | Parameter | Default | Description |
---|---|---|---|
string | event_name | Required parameter | The Event Name to unsubscribe |
function | callback? | nil | The callback function to unsubscribe |
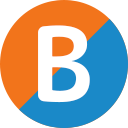
UnsubscribeRemote
Unsubscribes from all subscribed remote events in this Package with that event name, optionally passing the function to unsubscribe only that callback
Events.UnsubscribeRemote(event_name, callback?)