Base Entity
Entity is the base for all Classes, and all those entities share the same Methods and Events described in this page.
Classes that share methods and events from this Base Class: Billboard, Blueprint, Cable, Character, CharacterSimple, Decal, Gizmo, Grenade, Light, Melee, Particle, Player, Prop, SceneCapture, StaticMesh, Sound, TextRender, Trigger, VehicleWheeled, VehicleWater, Weapon, WebUI, Widget, Widget3D.
πΏ Static Functionsβ
The following functions are accessed statically using the specific class with a dot. Example: Character.GetAll()
.
Returns | Name | Description | |
---|---|---|---|
![]() | table of Base Entity | GetAll | Returns a table containing all Entities of the class this is called on |
![]() | Base Entity | GetByIndex | Returns a specific Entity of this class at an index |
![]() | integer | GetCount | Returns how many Entities of this class exist |
![]() | table of table | GetInheritedClasses | Gets a list of all directly inherited classes from this Class created with the Inheriting System |
![]() | iterator | GetPairs | Returns an iterator with all Entities of this class to be used with pairs() |
![]() | table or nil | GetParentClass | Gets the parent class if this Class was created with the Inheriting System |
![]() | table | Inherit | Inherits this class with the Inheriting System |
![]() | boolean | IsChildOf | Gets if this Class is child of another class if this Class was created with the Inheriting System |
![]() | function | Subscribe | Subscribes to an Event for all entities of this Class |
![]() | function | SubscribeRemote | Subscribes to a custom event called from server |
![]() | Unsubscribe | Unsubscribes all callbacks from this Event in this Class within this Package, or only the callback passed |
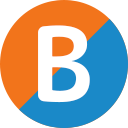
GetAll
Returns a table containing all Entities of the class this is called on
β Returns table of Base Entity (Copy of table containing all Entities).
local ret = Entity.GetAll()
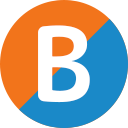
GetByIndex
Returns a specific Entity of this class at an index
β Returns Base Entity (Entity at index).
local ret = Entity.GetByIndex(index)
Type | Parameter | Default | Description |
---|---|---|---|
integer | index | Required parameter | The index of the Entity |
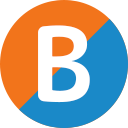
GetCount
Returns how many Entities of this class exist
β Returns integer (Number of Entities of this class).
local ret = Entity.GetCount()
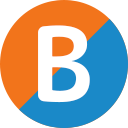
GetInheritedClasses
Gets a list of all directly inherited classes from this Class created with the Inheriting System
β Returns table of table (All children Classes).
local ret = Entity.GetInheritedClasses(recursively?)
Type | Parameter | Default | Description |
---|---|---|---|
boolean | recursively? | false | Returns all inherited children |
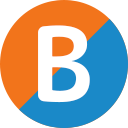
GetPairs
Returns an iterator with all Entities of this class to be used withpairs()
. This is a more performant method thanGetAll()
, as it will return the iterator to access the Entities directly instead of creating and returning a copy of the Entities table.
Note: Destroying Entities from inside aGetPairs()
loop will cause the iterable to change size during the process. If you want to loop-and-destroy, please useGetAll()
.
β Returns iterator (Iterator with all Entities of this class).
local ret = Entity.GetPairs()
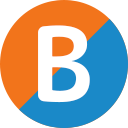
GetParentClass
Gets the parent class if this Class was created with the Inheriting System
β Returns table or nil (The parent class).
local ret = Entity.GetParentClass()
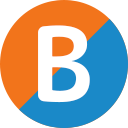
Inherit
Inherits this class with the Inheriting System
β Returns table (The new Class table).
local ret = Entity.Inherit(name, custom_values)
Type | Parameter | Default | Description |
---|---|---|---|
string | name | Required parameter | The name of the new Class |
table or nil | custom_values | Required parameter | An optional table with custom values to be set in the inherited class table |
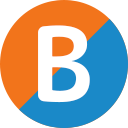
IsChildOf
Gets if this Class is child of another class if this Class was created with the Inheriting System
β Returns boolean.
local ret = Entity.IsChildOf(class)
Type | Parameter | Default | Description |
---|---|---|---|
table | class | Required parameter | The other class to check |
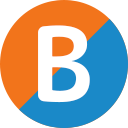
Subscribe
Subscribes to an Event for all entities of this Class
β Returns function (Callback that was passed (useful for unsubscribing later if your callback is an anonymous function)).
local ret = Entity.Subscribe(event_name, callback)
Type | Parameter | Default | Description |
---|---|---|---|
string | event_name | Required parameter | Name of the event to subscribe to |
function | callback | Required parameter | Function to call when the event is triggered |
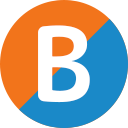
SubscribeRemote
Subscribes to a custom event called from server
β Returns function (Callback that was passed (useful for unsubscribing later if your callback is an anonymous function)).
local ret = Entity.SubscribeRemote(event_name, callback)
Type | Parameter | Default | Description |
---|---|---|---|
string | event_name | Required parameter | Name of the event to subscribe to |
function | callback | Required parameter | Function to call when the event is triggered |
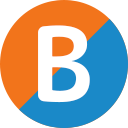
Unsubscribe
Unsubscribes all callbacks from this Event in this Class within this Package, or only the callback passed
Entity.Unsubscribe(event_name, callback?)
Type | Parameter | Default | Description |
---|---|---|---|
string | event_name | Required parameter | Name of the event to unsubscribe from |
function | callback? | nil | Optional callback to unsubscribe |
π¦ Functionsβ
Returns | Name | Description | |
---|---|---|---|
BroadcastRemoteEvent | Calls a custom remote event directly on this entity to all Players | ||
CallRemoteEvent | Calls a custom remote event directly on this entity to a specific Player | ||
CallRemoteEvent | Calls a custom remote event directly on this entity | ||
Destroy | Destroys this Entity | ||
table of string | GetAllValuesKeys | Gets a list of all values keys | |
![]() | table | GetClass | Gets the class of this entity |
![]() | integer | GetID | Gets the universal network ID of this Entity (same on both client and server) |
![]() | any | GetValue | Gets a Value stored on this Entity at the given key |
![]() | boolean | IsA | Recursively checks if this entity is inherited from a Class |
![]() | boolean | IsValid | Returns true if this Entity is valid (i.e. wasn't destroyed and points to a valid Entity) |
![]() | SetValue | Sets a Value in this Entity | |
![]() | function | Subscribe | Subscribes to an Event on this specific entity |
![]() | function | SubscribeRemote | Subscribes to a custom event called from server on this specific entity |
![]() | Unsubscribe | Unsubscribes all callbacks from this Event in this Entity within this Package, or only the callback passed |

BroadcastRemoteEvent
Calls a custom remote event directly on this entity to all Players
my_entity:BroadcastRemoteEvent(event_name, args...?)
Type | Parameter | Default | Description |
---|---|---|---|
string | event_name | Required parameter | The Event Name to trigger the event |
any | args...? | nil | Arguments to pass to the event |

CallRemoteEvent
Calls a custom remote event directly on this entity to a specific Player
my_entity:CallRemoteEvent(event_name, player, args...?)
Type | Parameter | Default | Description |
---|---|---|---|
string | event_name | Required parameter | The Event Name to trigger the event |
Player | player | Required parameter | The remote player to send this event |
any | args...? | nil | Arguments to pass to the event |

CallRemoteEvent
Calls a custom remote event directly on this entity
my_entity:CallRemoteEvent(event_name, args...?)
Type | Parameter | Default | Description |
---|---|---|---|
string | event_name | Required parameter | The Event Name to trigger the event |
any | args...? | nil | Arguments to pass to the event |

Destroy
Destroys this Entity
my_entity:Destroy()

GetAllValuesKeys
Gets a list of all values keys
β Returns table of string (a list with all values keys).
local ret = my_entity:GetAllValuesKeys()
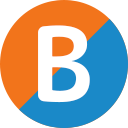
GetClass
Gets the class of this entity
β Returns table.
local ret = my_entity:GetClass()
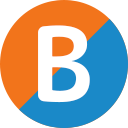
GetID
Gets the universal network ID of this Entity (same on both client and server)
β Returns integer.
local ret = my_entity:GetID()
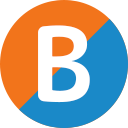
GetValue
Gets a Value stored on this Entity at the given key. Please refer to Entity Values for more information
β Returns any (Value at key or fallback if key doesn't exist).
local ret = my_entity:GetValue(key, fallback)
Type | Parameter | Default | Description |
---|---|---|---|
string | key | Required parameter | No description provided |
any | fallback | Required parameter | Fallback value if key doesn't exist |
See also GetAllValuesKeys, SetValue.
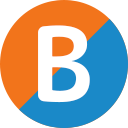
IsA
Recursively checks if this entity is inherited from a Class
β Returns boolean.
local ret = my_entity:IsA(class)
Type | Parameter | Default | Description |
---|---|---|---|
table | class | Required parameter | The Class |
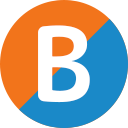
IsValid
Returns true if this Entity is valid (i.e. wasn't destroyed and points to a valid Entity)
β Returns boolean.
local ret = my_entity:IsValid()
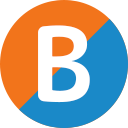
SetValue
Sets a value in this Entity, which can be accessed by any package (optionally sync on clients if called from server)
Please refer to Entity Values for more information
my_entity:SetValue(key, value, sync_on_clients?)
Type | Parameter | Default | Description |
---|---|---|---|
string | key | Required parameter | No description provided |
any | value | Required parameter | No description provided |
boolean | sync_on_clients? | false | Server side parameter, if enabled will sync this value with all clients |
See also GetAllValuesKeys, GetValue.
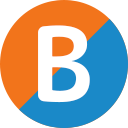
Subscribe
Subscribes to an Event on this specific entity
β Returns function (Callback that was passed (useful for unsubscribing later if your callback is an anonymous function)).
local ret = my_entity:Subscribe(event_name, callback)
Type | Parameter | Default | Description |
---|---|---|---|
string | event_name | Required parameter | Name of the event to subscribe to |
function | callback | Required parameter | Function to call when the event is triggered |
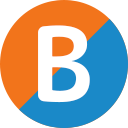
SubscribeRemote
Subscribes to a custom event called from server on this specific entity
β Returns function (Callback that was passed (useful for unsubscribing later if your callback is an anonymous function)).
local ret = my_entity:SubscribeRemote(event_name, callback)
Type | Parameter | Default | Description |
---|---|---|---|
string | event_name | Required parameter | Name of the event to subscribe to |
function | callback | Required parameter | Function to call when the event is triggered |
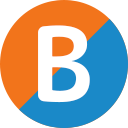
Unsubscribe
Unsubscribes all callbacks from this Event in this Entity within this Package, or only the callback passed
my_entity:Unsubscribe(event_name, callback?)
Type | Parameter | Default | Description |
---|---|---|---|
string | event_name | Required parameter | Name of the event to unsubscribe from |
function | callback? | nil | Optional callback to unsubscribe |
π Eventsβ
Name | Description | |
---|---|---|
![]() | ClassRegister | Triggered when a new Class is registered with the Inheriting System |
![]() | Destroy | Triggered when an Entity is destroyed |
![]() | Spawn | Triggered when an Entity is spawned/created |
![]() | ValueChange | Triggered when an Entity has a value changed with :SetValue() |
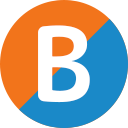
ClassRegister
Triggered when a new Class is registered with the Inheriting System
Entity.Subscribe("ClassRegister", function(class)
-- ClassRegister was called
end)
Type | Argument | Description |
---|---|---|
table | class | The inherited Class |
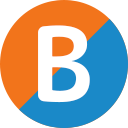
Destroy
Triggered when an Entity is destroyed
Entity.Subscribe("Destroy", function(self)
-- Destroy was called
end)
Type | Argument | Description |
---|---|---|
Base Entity | self | The Entity which that was destroyed |
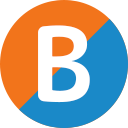
Spawn
Triggered when an Entity is spawned/created
Entity.Subscribe("Spawn", function(self)
-- Spawn was called
end)
Type | Argument | Description |
---|---|---|
Base Entity | self | The Entity that was spawned |
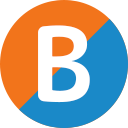
ValueChange
Triggered when an Entity has a value changed with :SetValue()
Entity.Subscribe("ValueChange", function(self, key, value)
-- ValueChange was called
end)
Type | Argument | Description |
---|---|---|
Base Entity | self | The Entity that just had a value changed |
string | key | The key used |
any | value | The value that was set |